使用C语言编写代码实现普通PC通过USB转485串口控制伺服电机转动
时间: 2023-12-31 19:05:50 浏览: 38
好的,下面是一份使用C语言编写的简单示例代码,实现了通过USB转485串口控制伺服电机转动的功能。需要注意的是,这份代码仅供参考,具体的实现方式可能会因伺服电机和USB转485串口设备的差异而有所不同。
```
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <unistd.h>
#include <errno.h>
#include <fcntl.h>
#include <termios.h>
#define BUF_SIZE 100
#define DEV_NAME "/dev/ttyUSB0" // USB转485串口设备名称
#define BAUD_RATE B9600 // 串口波特率
#define DATA_BITS CS8 // 数据位
#define STOP_BITS CSTOPB // 停止位
#define PARITY 0 // 校验位
#define SLAVE_ADDR 1 // 伺服电机从站地址
#define FUNC_CODE 6 // 控制命令的功能码
#define REG_ADDR 1 // 控制命令的寄存器地址
#define REG_VALUE 100 // 控制命令的寄存器值
// 计算ModBus-RTU协议的CRC校验码
unsigned short crc16(unsigned char *buf, int len) {
unsigned short crc = 0xFFFF;
for (int i = 0; i < len; i++) {
crc ^= buf[i];
for (int j = 0; j < 8; j++) {
if (crc & 0x0001) {
crc >>= 1;
crc ^= 0xA001;
} else {
crc >>= 1;
}
}
}
return crc;
}
// 发送控制命令到伺服电机
int send_cmd(int fd) {
unsigned char tx_buf[BUF_SIZE];
unsigned char rx_buf[BUF_SIZE];
int tx_len, rx_len;
// 构造控制命令的ModBus-RTU帧
tx_buf[0] = SLAVE_ADDR;
tx_buf[1] = FUNC_CODE;
tx_buf[2] = (REG_ADDR >> 8) & 0xFF;
tx_buf[3] = REG_ADDR & 0xFF;
tx_buf[4] = (REG_VALUE >> 8) & 0xFF;
tx_buf[5] = REG_VALUE & 0xFF;
// 计算CRC校验码
unsigned short crc = crc16(tx_buf, 6);
tx_buf[6] = crc & 0xFF;
tx_buf[7] = (crc >> 8) & 0xFF;
// 发送数据帧
tx_len = write(fd, tx_buf, 8);
if (tx_len != 8) {
printf("Error: Failed to send data frame! (errno = %d)\n", errno);
return -1;
}
// 等待接收响应数据帧
rx_len = read(fd, rx_buf, BUF_SIZE);
if (rx_len <= 0) {
printf("Error: Failed to receive response data frame! (errno = %d)\n", errno);
return -1;
}
// 解析响应数据帧
if (rx_buf[0] != SLAVE_ADDR || rx_buf[1] != FUNC_CODE || rx_buf[2] != (REG_ADDR >> 8) || rx_buf[3] != (REG_ADDR & 0xFF) || rx_buf[4] != (REG_VALUE >> 8) || rx_buf[5] != (REG_VALUE & 0xFF)) {
printf("Error: Invalid response data frame!\n");
return -1;
}
return 0;
}
int main() {
int fd;
struct termios options;
// 打开USB转485串口设备
fd = open(DEV_NAME, O_RDWR | O_NOCTTY);
if (fd < 0) {
printf("Error: Failed to open serial port %s! (errno = %d)\n", DEV_NAME, errno);
return -1;
}
// 配置串口通信参数
bzero(&options, sizeof(options));
options.c_cflag |= BAUD_RATE | DATA_BITS | STOP_BITS | PARITY;
tcsetattr(fd, TCSANOW, &options);
// 发送控制命令
if (send_cmd(fd) < 0) {
printf("Error: Failed to send control command!\n");
return -1;
}
// 关闭USB转485串口设备
close(fd);
return 0;
}
```
相关推荐
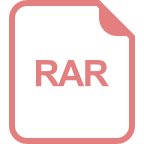
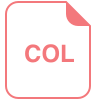
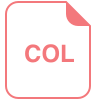
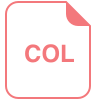
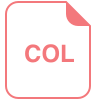
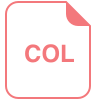









