springboot 实现雪花算法 完整代码
时间: 2023-05-28 17:06:56 浏览: 170
以下是一个完整的Spring Boot项目中实现雪花算法的代码示例:
```java
import org.springframework.stereotype.Component;
@Component
public class SnowflakeIdGenerator {
private final long twepoch = 1420041600000L;
private final long workerIdBits = 5L;
private final long datacenterIdBits = 5L;
private final long maxWorkerId = -1L ^ (-1L << workerIdBits);
private final long maxDatacenterId = -1L ^ (-1L << datacenterIdBits);
private final long sequenceBits = 12L;
private final long workerIdShift = sequenceBits;
private final long datacenterIdShift = sequenceBits + workerIdBits;
private final long timestampLeftShift = sequenceBits + workerIdBits + datacenterIdBits;
private final long sequenceMask = -1L ^ (-1L << sequenceBits);
private long workerId;
private long datacenterId;
private long sequence = 0L;
private long lastTimestamp = -1L;
public SnowflakeIdGenerator() {
this.workerId = 0L;
this.datacenterId = 0L;
}
public synchronized long nextId() {
long timestamp = timeGen();
if (timestamp < lastTimestamp) {
throw new RuntimeException("Clock moved backwards. Refusing to generate id");
}
if (lastTimestamp == timestamp) {
sequence = (sequence + 1) & sequenceMask;
if (sequence == 0) {
timestamp = tilNextMillis(lastTimestamp);
}
} else {
sequence = 0L;
}
lastTimestamp = timestamp;
return ((timestamp - twepoch) << timestampLeftShift) |
(datacenterId << datacenterIdShift) |
(workerId << workerIdShift) |
sequence;
}
private long tilNextMillis(long lastTimestamp) {
long timestamp = timeGen();
while (timestamp <= lastTimestamp) {
timestamp = timeGen();
}
return timestamp;
}
private long timeGen() {
return System.currentTimeMillis();
}
}
```
在上述代码中,我们定义了一个名为SnowflakeIdGenerator的组件,用于生成雪花算法的ID。在组件的构造函数中,我们初始化了workerId和datacenterId的值,并定义了一些常量,如twepoch(起始时间戳)、workerIdBits、datacenterIdBits等。在nextId()方法中,我们利用当前时间戳、workerId、datacenterId和序列号生成了一个唯一的ID,并返回给调用方。注意到这里使用了synchronized关键字来确保线程安全。
使用该组件非常简单,只需在需要生成ID的地方注入该组件即可。例如,在一个Controller中使用该组件生成ID:
```java
@RestController
@RequestMapping("/api")
public class MyController {
@Autowired
private SnowflakeIdGenerator idGenerator;
@PostMapping("/create")
public ResponseEntity<String> create() {
long id = idGenerator.nextId();
return ResponseEntity.ok("Generated ID: " + id);
}
}
```
当然,该组件还可以进一步优化,例如可以将workerId和datacenterId从配置文件中读取,以便在不同的环境中使用不同的值。
相关推荐
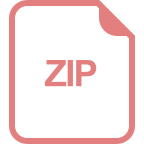
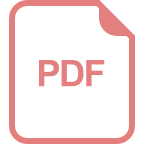














