以上述代码为例,用C语言实现多文件编程:在工程中添加头文件1.h,在该文件中声明两个函数,int fun(int n);void sort(int a[],int n);保存。在工程中添加新文件1.cpp,将fun()函数和sort()函数的定义移至1.cpp,并在该文件的前面包含头文件1.h(#include”1.h”)。在主函数的文件中的前面也包含1.h头文件。然后编译运行该工程,观察输出结果。
时间: 2024-02-11 21:07:28 浏览: 19
以下是多文件编程的C语言代码实现:
头文件 `1.h`:
```c
#ifndef _1_H_
#define _1_H_
void sort(int a[], int n);
int fun(int n);
#endif
```
源文件 `1.cpp`:
```c
#include "1.h"
// 从大到小排序数组
void sort(int a[], int n) {
for (int i = 0; i < n; i++) {
for (int j = i+1; j < n; j++) {
if (a[i] < a[j]) {
int temp = a[i];
a[i] = a[j];
a[j] = temp;
}
}
}
}
// 将自然数拆分成单个数字并排序成最大数
int fun(int n) {
int a[4];
int i = 0;
while (n > 0) {
a[i++] = n % 10;
n /= 10;
}
sort(a, 4);
return a[0]*1000 + a[1]*100 + a[2]*10 + a[3];
}
```
主程序文件 `main.c`:
```c
#include <stdio.h>
#include "1.h"
int main() {
int num;
printf("请输入一个4位自然数:");
scanf("%d", &num);
int result = fun(num);
printf("各位数字组成的最大数为:%d\n", result);
return 0;
}
```
程序中定义了头文件 `1.h` 和源文件 `1.cpp`,其中头文件中声明了两个函数 `sort()` 和 `fun()`,源文件中定义了这两个函数的具体实现。在主程序文件 `main.c` 中,我们通过 `#include "1.h"` 的方式将头文件包含进来,然后调用 `fun()` 函数得到各位数字组成的最大数,并输出到屏幕上。
编译命令:`gcc main.c 1.cpp -o result`,运行命令:`./result`。
相关推荐
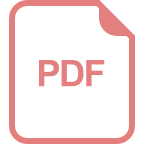
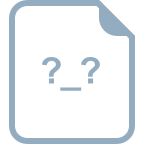
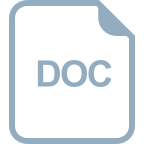














