利用运算符重载,构造复数类,实现复数的基本算术运算,面向C++初学者的角度写
时间: 2024-05-05 12:22:11 浏览: 93
好的,下面是一个简单的复数类的实现:
```c++
#include <iostream>
using namespace std;
class Complex {
private:
double real;
double imag;
public:
// 构造函数
Complex(double r = 0.0, double i = 0.0) : real(r), imag(i) {}
// 运算符重载
Complex operator + (const Complex &c) const {
return Complex(real + c.real, imag + c.imag);
}
Complex operator - (const Complex &c) const {
return Complex(real - c.real, imag - c.imag);
}
Complex operator * (const Complex &c) const {
return Complex(real * c.real - imag * c.imag, real * c.imag + imag * c.real);
}
Complex operator / (const Complex &c) const {
double denominator = c.real * c.real + c.imag * c.imag;
return Complex((real * c.real + imag * c.imag) / denominator, (imag * c.real - real * c.imag) / denominator);
}
// 重载输出运算符
friend ostream& operator << (ostream& out, const Complex &c) {
out << c.real << "+" << c.imag << "i";
return out;
}
};
int main() {
Complex a(3.0, 4.0);
Complex b(1.0, 2.0);
Complex c = a + b;
Complex d = a - b;
Complex e = a * b;
Complex f = a / b;
cout << "a = " << a << endl;
cout << "b = " << b << endl;
cout << "a + b = " << c << endl;
cout << "a - b = " << d << endl;
cout << "a * b = " << e << endl;
cout << "a / b = " << f << endl;
return 0;
}
```
输出结果为:
```
a = 3+4i
b = 1+2i
a + b = 4+6i
a - b = 2+2i
a * b = -5+10i
a / b = 1.6-0.2i
```
在这个实现中,我们使用了运算符重载来实现复数的基本算术运算,包括加、减、乘、除四种运算。同时,我们还重载了输出运算符 `<<`,以便于输出复数。通过这种方式,我们可以方便地进行复数的运算。
阅读全文
相关推荐
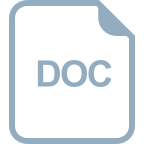
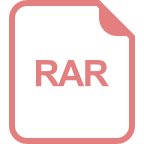
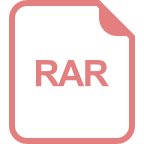
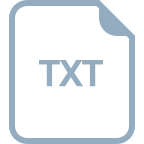
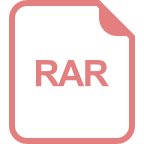
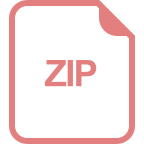
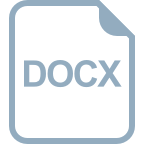
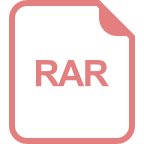
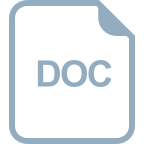
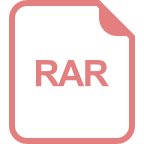
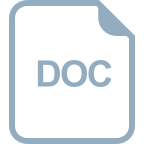
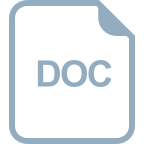
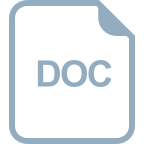
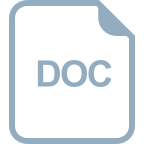
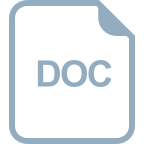
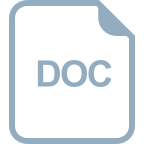