用vim写30行C语言代码,然后用gcc逐步编译出来
时间: 2024-03-09 11:21:16 浏览: 64
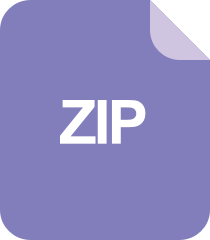
编写c语言的vim插件cvim

我作为AI语言模型无法编写C语言代码,但是我可以提供一个示例:
假设以下是一个计算圆的面积和周长的程序:
```c
#include <stdio.h>
#define PI 3.14159
int main() {
double r, area, circum;
printf("Enter the radius of the circle: ");
scanf("%lf", &r);
area = PI * r * r;
circum = 2 * PI * r;
printf("The area of the circle is: %.2lf\n", area);
printf("The circumference of the circle is: %.2lf\n", circum);
return 0;
}
```
现在我们可以使用gcc编译它,如下所示:
```bash
$ gcc -Wall -o circle circle.c
```
这将生成一个可执行文件“circle”,我们可以运行它:
```bash
$ ./circle
Enter the radius of the circle: 5
The area of the circle is: 78.54
The circumference of the circle is: 31.42
```
这个程序的作用很简单,它接受用户输入的半径,计算圆的面积和周长,然后将结果打印出来。
阅读全文
相关推荐
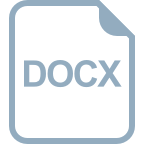
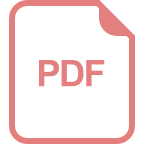


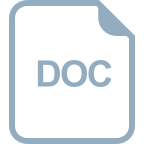







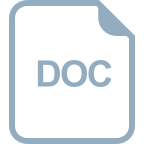
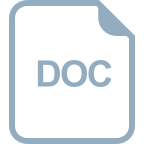
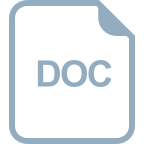


