用java实现hbase数据库的增删改查
时间: 2023-09-11 15:12:09 浏览: 133
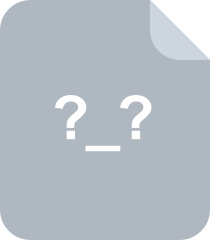
java调用hbase实现数据库的增删改查
好的,我可以帮您回答这个问题。下面是使用 Java 操作 HBase 数据库的增删改查示例代码:
1. 导入必要的包
```
import org.apache.hadoop.conf.Configuration;
import org.apache.hadoop.hbase.Cell;
import org.apache.hadoop.hbase.CellUtil;
import org.apache.hadoop.hbase.HBaseConfiguration;
import org.apache.hadoop.hbase.TableName;
import org.apache.hadoop.hbase.client.*;
import org.apache.hadoop.hbase.util.Bytes;
```
2. 创建 HBase 配置对象
```
Configuration configuration = HBaseConfiguration.create();
configuration.set("hbase.zookeeper.quorum", "localhost"); // HBase 的 ZooKeeper 地址
configuration.set("hbase.zookeeper.property.clientPort", "2181"); // HBase 的 ZooKeeper 端口号
```
3. 创建 HBase 连接对象和表对象
```
Connection connection = ConnectionFactory.createConnection(configuration);
Table table = connection.getTable(TableName.valueOf("test_table")); // test_table 是表名
```
4. 插入数据
```
Put put = new Put(Bytes.toBytes("row1")); // row1 是行键名
put.addColumn(Bytes.toBytes("cf"), Bytes.toBytes("col1"), Bytes.toBytes("value1")); // cf 是列族名,col1 是列名,value1 是值
table.put(put);
```
5. 查询数据
```
Get get = new Get(Bytes.toBytes("row1")); // row1 是行键名
Result result = table.get(get);
for (Cell cell : result.listCells()) {
String cf = Bytes.toString(CellUtil.cloneFamily(cell)); // 获取列族名
String col = Bytes.toString(CellUtil.cloneQualifier(cell)); // 获取列名
String value = Bytes.toString(CellUtil.cloneValue(cell)); // 获取值
System.out.println(cf + ":" + col + "=" + value);
}
```
6. 修改数据
```
Put put = new Put(Bytes.toBytes("row1")); // row1 是行键名
put.addColumn(Bytes.toBytes("cf"), Bytes.toBytes("col1"), Bytes.toBytes("new_value")); // cf 是列族名,col1 是列名,new_value 是新的值
table.put(put);
```
7. 删除数据
```
Delete delete = new Delete(Bytes.toBytes("row1")); // row1 是行键名
delete.addColumn(Bytes.toBytes("cf"), Bytes.toBytes("col1")); // cf 是列族名,col1 是列名
table.delete(delete);
```
以上就是使用 Java 操作 HBase 数据库的增删改查示例代码,希望对您有所帮助。
阅读全文
相关推荐

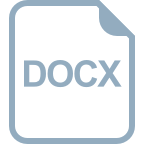
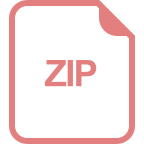
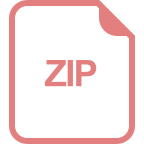
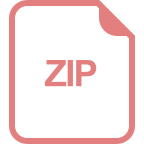
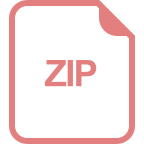
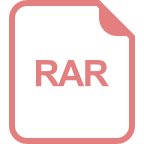
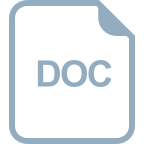
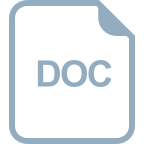

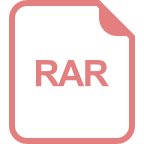
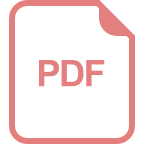
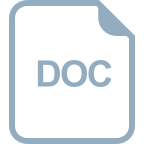
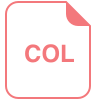
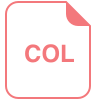