返回树形结构给前端的代码
时间: 2023-08-09 21:06:07 浏览: 95
好的,如果您需要将构建好的树形结构返回给前端,可以使用Java中的JSON库将树形结构转换为JSON格式。下面是一个简单的示例代码:
首先,我们需要在pom.xml中引入json库的依赖:
```
<dependency>
<groupId>com.google.code.gson</groupId>
<artifactId>gson</artifactId>
<version>2.8.6</version>
</dependency>
```
然后,我们需要修改TreeNode类,添加一个toJson方法,将树形结构转换为JSON格式:
```
import com.google.gson.Gson;
import com.google.gson.GsonBuilder;
import java.util.HashMap;
import java.util.Map;
public class TreeNode {
private String name;
private Map<String, TreeNode> children;
public TreeNode(String name) {
this.name = name;
this.children = new HashMap<>();
}
public String getName() {
return name;
}
public Map<String, TreeNode> getChildren() {
return children;
}
public void addChild(TreeNode child) {
this.children.put(child.getName(), child);
}
public String toJson() {
Gson gson = new GsonBuilder().setPrettyPrinting().create();
return gson.toJson(this);
}
}
```
上面的代码中,toJson方法使用了Gson库将树形结构转换为JSON格式,并使用了setPrettyPrinting方法进行了格式化。
接下来,我们可以在Main类中调用toJson方法将树形结构转换为JSON格式,并返回给前端。下面是一个简单的示例代码:
```
import java.util.*;
public class Main {
public static void main(String[] args) {
List<String> paths = Arrays.asList(
"通道1",
"通道1.设备1",
"通道1.设备1.DECNO",
"通道1.设备1.DECSTATUS",
"通道1.设备1.TAG2",
"通道1.设备1.technology",
"通道1.设备1.TIME",
"通道1.设备1.v1",
"通道1.设备1.v2",
"通道2",
"通道2.设备1",
"通道2.设备1.TAG1",
"西门子PLC",
"西门子PLC.s7-300"
);
TreeNode root = TreeNode.buildTree(paths);
String json = root.toJson();
System.out.println(json);
}
}
```
运行上面的代码,可以得到如下的JSON输出结果:
```
{
"name": "",
"children": {
"通道1": {
"name": "通道1",
"children": {
"设备1": {
"name": "设备1",
"children": {
"DECNO": {
"name": "DECNO",
"children": {}
},
"DECSTATUS": {
"name": "DECSTATUS",
"children": {}
},
"TAG2": {
"name": "TAG2",
"children": {}
},
"technology": {
"name": "technology",
"children": {}
},
"TIME": {
"name": "TIME",
"children": {}
},
"v1": {
"name": "v1",
"children": {}
},
"v2": {
"name": "v2",
"children": {}
}
}
}
}
},
"通道2": {
"name": "通道2",
"children": {
"设备1": {
"name": "设备1",
"children": {
"TAG1": {
"name": "TAG1",
"children": {}
}
}
}
}
},
"西门子PLC": {
"name": "西门子PLC",
"children": {
"s7-300": {
"name": "s7-300",
"children": {}
}
}
}
}
}
```
需要注意的是,上面的代码中只是简单地将树形结构转换为JSON格式,如果您需要进行更复杂的操作,可以根据实际情况进行扩展。
阅读全文
相关推荐





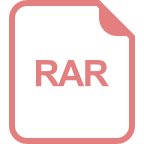
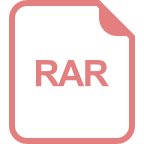








