将两个字符串连接起来,不用strcat函数
时间: 2023-06-07 15:09:22 浏览: 67
你好,关于你的问题,可以使用以下代码将两个字符串连接起来:
```c++
#include <iostream>
#include <cstring>
using namespace std;
int main() {
char str1[20] = "Hello";
char str2[20] = " World!";
int i = 0, j = 0;
while (str1[i] != '\0') {
i++;
}
while (str2[j] != '\0') {
str1[i] = str2[j];
i++;
j++;
}
str1[i] = '\0'; // 添加字符串结束符
cout << str1 << endl;
return 0;
}
```
上述代码中,首先将指针 `i` 和 `j` 初始化为 0,然后使用 `while` 循环找到字符串 `str1` 的末尾位置。接着使用另一个 `while` 循环,逐个将 `str2` 中的字符添加到 `str1` 的末尾,直到 `str2` 的末尾位置。最后,在 `str1` 的末尾添加字符串结束符 `\0`,以表示字符串的结束。最终,使用 `cout` 输出拼接后的字符串 `str1`。
希望能解答您的问题。
相关问题
将两个字符串连接起来不用strcat函数
### 回答1:
可以使用循环遍历两个字符串,将第一个字符串的每个字符依次存入一个新的字符串中,然后再将第二个字符串的每个字符依次存入新的字符串中。最终得到的新字符串即为两个字符串的连接结果。具体实现代码如下:
```python
def concat_str(str1, str2):
new_str = ''
for ch in str1:
new_str += ch
for ch in str2:
new_str += ch
return new_str
```
```java
public static String concatStr(String str1, String str2) {
StringBuilder sb = new StringBuilder();
for (int i = ; i < str1.length(); i++) {
sb.append(str1.charAt(i));
}
for (int i = ; i < str2.length(); i++) {
sb.append(str2.charAt(i));
}
return sb.toString();
}
```
```c++
#include <iostream>
using namespace std;
string concat_str(string str1, string str2) {
string new_str = "";
for (int i = ; i < str1.length(); i++) {
new_str += str1[i];
}
for (int i = ; i < str2.length(); i++) {
new_str += str2[i];
}
return new_str;
}
int main() {
string str1 = "hello";
string str2 = "world";
string result = concat_str(str1, str2);
cout << result << endl; // 输出 "helloworld"
return ;
}
```
### 回答2:
字符串连接操作是在编程中经常用到的操作,通常情况下我们可以通过strcat函数来实现字符串连接。但是有时候,我们需要自己手动实现字符串连接,并且不使用strcat函数。下面我将详细讲解一下这个问题的解决方案。
实现字符串连接,最基本的操作就是将一个字符串中的每个字符都复制到目标字符串中。因此,我们可以通过for循环来实现字符串的连接。具体实现方法可以按照以下步骤:
1. 先计算出目标字符串的长度。
我们可以利用strlen函数来计算原字符串的长度,并且根据字符串连接的方法,可以得出目标字符串的长度,即两个字符串长度的和。
2. 为目标字符串申请空间。
根据目标字符串的长度,我们可以使用malloc函数来为目标字符串申请空间。
3. 复制原字符串到目标字符串中。
我们利用for循环来遍历原字符串中的每个字符,并且将其依次复制到目标字符串中。
4. 复制第二个字符串到目标字符串中
使用指针运算,将第二个字符串中的字符也复制到目标字符串中。
5. 添加结尾标识符’\0’
将最后一个位置添加上结尾标识符,即'0',使目标字符串具有正常存储形式。
6. 输出结果
最后,我们可以输出字符串连接的结果。
以上就是基于for循环手动实现字符串连接的步骤,这个方法虽然较为繁琐,但也是十分实用的。通过这种方法实现字符串连接可以提高程序的灵活性,同时也有利于理解字符串的本质。
### 回答3:
字符串连接是日常开发中经常遇到的问题,常用的方法是使用C语言中的strcat函数。不过,如果不想使用strcat函数,也有其他的方法可以实现字符串连接。
第一种方法是使用for循环遍历两个字符串,将第一个字符串的字符逐一添加到新的字符串中,然后再将第二个字符串的字符也逐一添加到新的字符串中。具体的实现过程如下所示:
```
#include <stdio.h>
int main() {
char s1[] = "abc";
char s2[] = "def";
char s3[7]; // 新的字符串
int i=0, j=0;
// 复制第一个字符串
while (s1[i]) {
s3[i] = s1[i];
i++;
}
// 复制第二个字符串
while (s2[j]) {
s3[i+j] = s2[j];
j++;
}
s3[i+j] = '\0'; // 添加字符串结束符
printf("连接后的字符串为: %s\n", s3);
return 0;
}
```
第二种方法是使用指针。将新的字符串的首地址指向第一个字符串,然后以此向后遍历,直到遇到第一个字符串的结束符,再将指针指向第二个字符串的首地址,重复遍历,直到遇到第二个字符串的结束符。具体的实现过程如下所示:
```
#include <stdio.h>
int main() {
char s1[] = "abc";
char s2[] = "def";
char s3[7]; // 新的字符串
char *p = s3; // 指针指向s3的首地址
// 复制第一个字符串
while (*p++ = *s1++) {} // 注意这里的++是先执行,后赋值(从左到右),遇到\0跳出循环
// 复制第二个字符串
while (*p++ = *s2++) {} // 同上
printf("连接后的字符串为: %s\n", s3);
return 0;
}
```
以上是两种实现字符串连接的方法,大家可以根据项目需求选择其中的一种方法进行使用。
将两个字符串连接起来不用strcat
可以使用 "+" 操作符将两个字符串连接起来,例如:
```
string1 = "Hello"
string2 = " World"
result = string1 + string2
print(result)
# 输出 "Hello World"
```
也可以使用字符串格式化字符串,例如:
```
string1 = "Hello"
string2 = " World"
result = "%s%s"%(string1,string2)
print(result)
# 输出 "Hello World"
```
或者使用join函数
```
string1 = "Hello"
string2 = " World"
result = " ".join([string1, string2])
print(result)
# 输出 "Hello World"
```
相关推荐
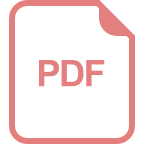
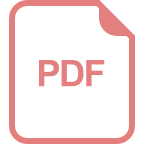
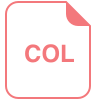
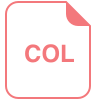
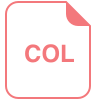
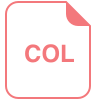
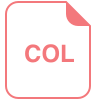







