编写加减乘除4个自定义函数add,sub,mul,div,在主函数中调用这4个函数去完成以下功能: (1)随机生成5道四则运算题,题目的类型自加,减,乘,除中任意混合出题(即5道题目中有各种类型的题目),每个题目的2个整数是20以内的随机数,题目显示在屏幕上,由用户输入答案(如下图所示)。 (2)程序判断用户输入的答案是否正确,统计答题的正确率。运行结束时,询问是否继续练习,如果回答“Y”则继续进行练习,如果回答“N”则退出练习。 (3)把每次所做的题目和答题的准确率输出在屏幕上,同时也把每次的运行结果保存到result.txt文本文件中。
时间: 2024-03-13 16:47:44 浏览: 17
好的,我会根据你的要求进行回答。以下是代码实现:
```python
import random
def add(a, b):
return a + b
def sub(a, b):
return a - b
def mul(a, b):
return a * b
def div(a, b):
return a / b
def generate_question():
operations = ['+', '-', '*', '/']
a = random.randint(1, 20)
b = random.randint(1, 20)
operation = random.choice(operations)
if operation == '/' and a % b != 0:
b = random.randint(1, a)
question = f'{a} {operation} {b} = '
answer = ''
if operation == '+':
answer = add(a, b)
elif operation == '-':
answer = sub(a, b)
elif operation == '*':
answer = mul(a, b)
elif operation == '/':
answer = div(a, b)
return question, answer
def main():
correct_count = 0
total_count = 0
while True:
questions = []
answers = []
for i in range(5):
question, answer = generate_question()
questions.append(question)
answers.append(answer)
print(question, end='')
user_answer = input().strip()
if user_answer == str(answer):
correct_count += 1
total_count += 1
accuracy = correct_count / total_count
print('准确率:{:.2%}'.format(accuracy))
with open('result.txt', 'a') as f:
f.write(f'题目:{str(questions)}\n')
f.write(f'答案:{str(answers)}\n')
f.write(f'准确率:{accuracy:.2%}\n')
option = input('是否继续练习?(Y/N)').strip().lower()
if option == 'n':
break
if __name__ == '__main__':
main()
```
在这个程序中,我们定义了4个自定义函数`add`、`sub`、`mul`、`div`,用于进行加减乘除运算。`generate_question`函数用于随机生成一个运算题目,并返回题目和答案。`main`函数用于进行主要流程的控制,包括随机生成5道题目,用户输入答案,判断答案是否正确,输出准确率,保存结果到文件,询问是否继续练习等。程序的运行结果和保存的结果会保存在`result.txt`文件中。
相关推荐
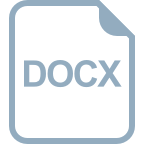
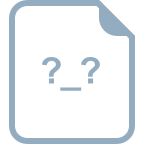














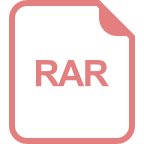