RSA算法数字签名C语言编程实现
时间: 2023-07-26 12:28:58 浏览: 231
RSA算法数字签名C语言编程实现示例:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <openssl/rsa.h>
#include <openssl/pem.h>
#include <openssl/err.h>
#define KEY_LENGTH 2048
#define BLOCK_SIZE KEY_LENGTH/8-11
int main(){
RSA *keypair;
unsigned char plain_text[100] = "Hello, RSA!";
unsigned char cipher_text[KEY_LENGTH/8];
unsigned char decrypted_text[KEY_LENGTH/8];
unsigned char signature[KEY_LENGTH/8];
unsigned int signature_len;
int decrypted_len;
// 生成 RSA 密钥对
keypair = RSA_generate_key(KEY_LENGTH, RSA_F4, NULL, NULL);
// 使用私钥签名
if (RSA_sign(NID_sha256, plain_text, strlen(plain_text), signature, &signature_len, keypair) != 1) {
fprintf(stderr, "RSA sign error!\n");
RSA_free(keypair);
return -1;
}
// 使用公钥验签
if (RSA_verify(NID_sha256, plain_text, strlen(plain_text), signature, signature_len, keypair) != 1) {
fprintf(stderr, "RSA verify error!\n");
RSA_free(keypair);
return -1;
}
// 使用公钥加密
int cipher_len = RSA_public_encrypt(strlen(plain_text), plain_text, cipher_text, keypair, RSA_PKCS1_PADDING);
if (cipher_len == -1) {
fprintf(stderr, "RSA public encrypt error!\n");
RSA_free(keypair);
return -1;
}
printf("Cipher text: ");
for (int i = 0; i < cipher_len; i++) {
printf("%02x", cipher_text[i]);
}
printf("\n");
// 使用私钥解密
decrypted_len = RSA_private_decrypt(cipher_len, cipher_text, decrypted_text, keypair, RSA_PKCS1_PADDING);
if (decrypted_len == -1) {
fprintf(stderr, "RSA private decrypt error!\n");
RSA_free(keypair);
return -1;
}
decrypted_text[decrypted_len] = '\0';
printf("Decrypted text: %s\n", decrypted_text);
RSA_free(keypair);
return 0;
}
```
需要注意的是,这里用到了 OpenSSL 库,需要在编译时链接该库,同时需要在代码中包含相关的头文件。此外,为了方便,这里的签名、验签、加密、解密都是在同一个程序中实现,实际应用中可能需要拆分成不同的模块。
阅读全文
相关推荐
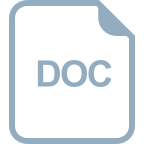
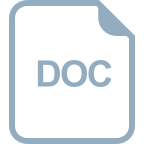
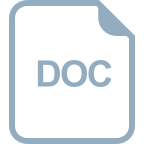
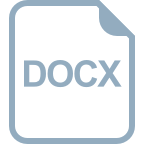
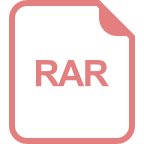
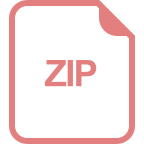
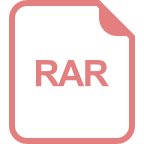
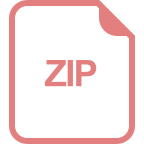
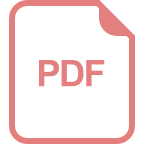
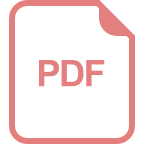
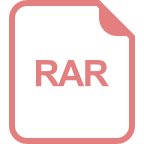
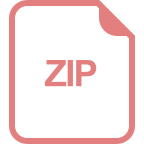
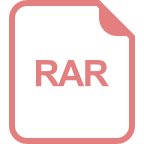
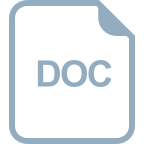
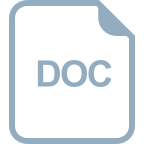


