c语言实现学生成绩管理系统
时间: 2023-07-13 15:37:28 浏览: 117
学生成绩管理系统可以通过 C 语言来实现。主要需要实现以下几个功能:
1. 学生信息的录入和存储:可以定义一个结构体用来存储学生信息,包括学号、姓名、性别、出生日期等,然后通过文件操作将学生信息保存到文件中。
2. 成绩的录入和存储:同样可以定义一个结构体来存储学生的成绩信息,包括科目名称、分数等,然后将成绩信息与学生信息关联起来,保存到文件中。
3. 成绩的查询:可以根据学号或姓名等关键字查询学生的成绩信息,然后将查询结果在屏幕上输出。
4. 成绩的统计:可以统计某一门或所有科目的平均成绩、最高分、最低分等数据,并将统计结果在屏幕上输出。
下面是一个简单的学生成绩管理系统的代码示例:
```
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define MAX_STUDENT_NUM 100
#define MAX_SUBJECT_NUM 5
typedef struct {
char id[20]; // 学号
char name[20]; // 姓名
char gender[10]; // 性别
char birthdate[20]; // 出生日期
} Student;
typedef struct {
char subject[20]; // 科目名称
int score; // 分数
} Score;
typedef struct {
Student student; // 学生信息
Score score[MAX_SUBJECT_NUM]; // 学生的成绩信息
} Record;
Record records[MAX_STUDENT_NUM]; // 所有学生的信息和成绩数据
int num_records = 0; // 学生数量
// 将学生信息和成绩信息保存到文件中
void save_records() {
FILE* fp = fopen("data.txt", "w");
if (fp == NULL) {
printf("Failed to open file for writing.\n");
return;
}
for (int i = 0; i < num_records; i++) {
fprintf(fp, "%s %s %s %s\n", records[i].student.id, records[i].student.name,
records[i].student.gender, records[i].student.birthdate);
for (int j = 0; j < MAX_SUBJECT_NUM; j++) {
if (strlen(records[i].score[j].subject) == 0) {
break;
}
fprintf(fp, "%s %d ", records[i].score[j].subject, records[i].score[j].score);
}
fprintf(fp, "\n");
}
fclose(fp);
}
// 从文件中读取学生信息和成绩信息
void load_records() {
FILE* fp = fopen("data.txt", "r");
if (fp == NULL) {
printf("Failed to open file for reading.\n");
return;
}
while (!feof(fp)) {
char line[1024];
Record record;
if (fgets(line, 1024, fp) == NULL) {
break;
}
sscanf(line, "%s %s %s %s", record.student.id, record.student.name,
record.student.gender, record.student.birthdate);
for (int j = 0; j < MAX_SUBJECT_NUM; j++) {
if (fscanf(fp, "%s %d", record.score[j].subject, &record.score[j].score) == 2) {
continue;
}
break;
}
records[num_records++] = record;
}
fclose(fp);
}
// 添加一条学生记录
void add_record() {
Record record;
printf("Please input student ID: ");
scanf("%s", record.student.id);
printf("Please input student name: ");
scanf("%s", record.student.name);
printf("Please input student gender: ");
scanf("%s", record.student.gender);
printf("Please input student birthdate: ");
scanf("%s", record.student.birthdate);
for (int i = 0; i < MAX_SUBJECT_NUM; i++) {
printf("Please input score for subject %d: ", i+1);
scanf("%s %d", record.score[i].subject, &record.score[i].score);
if (strlen(record.score[i].subject) == 0) {
break;
}
}
records[num_records++] = record;
save_records();
}
// 查询学生记录
void query_record() {
char keyword[20];
printf("Please input the keyword to search: ");
scanf("%s", keyword);
printf("Results:\n");
for (int i = 0; i < num_records; i++) {
if (strstr(records[i].student.id, keyword) != NULL ||
strstr(records[i].student.name, keyword) != NULL) {
printf("Student ID: %s, Name: %s\n", records[i].student.id, records[i].student.name);
for (int j = 0; j < MAX_SUBJECT_NUM; j++) {
if (strlen(records[i].score[j].subject) == 0) {
break;
}
printf("Subject: %s, Score: %d\n", records[i].score[j].subject, records[i].score[j].score);
}
}
}
}
// 统计成绩信息
void stat_score() {
char subject[20];
printf("Please input the subject to stat: ");
scanf("%s", subject);
int count = 0;
int total = 0;
int max_score = 0;
int min_score = 100;
for (int i = 0; i < num_records; i++) {
for (int j = 0; j < MAX_SUBJECT_NUM; j++) {
if (strcmp(records[i].score[j].subject, subject) == 0) {
count++;
total += records[i].score[j].score;
if (records[i].score[j].score > max_score) {
max_score = records[i].score[j].score;
}
if (records[i].score[j].score < min_score) {
min_score = records[i].score[j].score;
}
}
}
}
printf("Total count: %d\n", count);
printf("Average score: %.2f\n", count > 0 ? (float)total / count : 0);
printf("Max score: %d\n", max_score);
printf("Min score: %d\n", min_score);
}
int main() {
load_records();
while (1) {
int choice;
printf("Please select an operation:\n");
printf("1. Add record\n");
printf("2. Query record\n");
printf("3. Stat score\n");
printf("4. Exit\n");
printf("Your choice: ");
scanf("%d", &choice);
switch (choice) {
case 1:
add_record();
break;
case 2:
query_record();
break;
case 3:
stat_score();
break;
case 4:
return 0;
default:
printf("Invalid choice.\n");
}
}
}
```
以上是一个简单的学生成绩管理系统的 C 语言代码示例,其中使用了文件操作和结构体等 C 语言的基础知识。
阅读全文
相关推荐
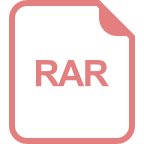
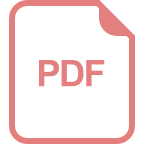
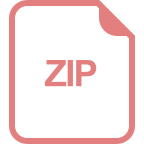
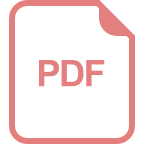
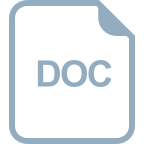
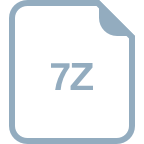
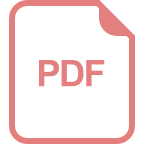
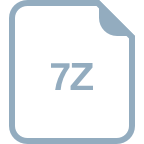
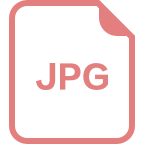