C#WinForm用PrintDialog 控件和三个赋值label1、label2、label3控件设置,实现label1、label2和label3控件可调整字体大小,可任意移动位置,然后通过按钮实现打印
时间: 2024-02-25 21:51:30 浏览: 132
好的,让我来解答你的问题。
首先,你需要在 C#WinForm 应用程序中添加一个 PrintDialog 控件和三个 Label 控件。然后,你可以在代码中使用以下方法设置 Label 控件的字体大小和位置:
```csharp
// 设置 Label 控件的字体大小
label1.Font = new Font("Arial", 12);
label2.Font = new Font("Arial", 12);
label3.Font = new Font("Arial", 12);
// 设置 Label 控件的位置
label1.Location = new Point(50, 50);
label2.Location = new Point(50, 100);
label3.Location = new Point(50, 150);
```
接下来,你需要实现 Label 控件的移动和调整字体大小的功能。你可以使用 MouseDown、MouseMove 和 MouseUp 事件来实现 Label 控件的移动,使用 MouseWheel 事件来实现 Label 控件的字体大小调整,如下所示:
```csharp
// Label 控件的 MouseDown 事件
private void label_MouseDown(object sender, MouseEventArgs e)
{
if (e.Button == MouseButtons.Left)
{
Label label = sender as Label;
label.Tag = new Point(e.X, e.Y);
}
}
// Label 控件的 MouseMove 事件
private void label_MouseMove(object sender, MouseEventArgs e)
{
if (e.Button == MouseButtons.Left)
{
Label label = sender as Label;
Point tag = (Point)label.Tag;
label.Left += e.X - tag.X;
label.Top += e.Y - tag.Y;
}
}
// Label 控件的 MouseUp 事件
private void label_MouseUp(object sender, MouseEventArgs e)
{
if (e.Button == MouseButtons.Left)
{
Label label = sender as Label;
label.Tag = null;
}
}
// Label 控件的 MouseWheel 事件
private void label_MouseWheel(object sender, MouseEventArgs e)
{
Label label = sender as Label;
float size = label.Font.Size + e.Delta / 120;
label.Font = new Font(label.Font.FontFamily, size, label.Font.Style);
}
```
在上面的代码中,label_MouseDown、label_MouseMove 和 label_MouseUp 方法实现 Label 控件的移动功能,label_MouseWheel 方法实现 Label 控件的字体大小调整功能。
最后,你可以创建一个按钮,并在按钮的 Click 事件中编写以下代码来打印 Label 控件:
```csharp
private void printButton_Click(object sender, EventArgs e)
{
// 显示打印对话框
if (printDialog1.ShowDialog() == DialogResult.OK)
{
// 创建一个 PrintDocument 对象
PrintDocument pd = new PrintDocument();
// 设置 PrintDocument 的打印页面
pd.PrintPage += new PrintPageEventHandler(pd_PrintPage);
// 将 PrintDocument 对象传递给 PrintDialog 控件,并启动打印操作
printDialog1.Document = pd;
pd.Print();
}
}
private void pd_PrintPage(object sender, PrintPageEventArgs e)
{
// 设置 Label 控件的位置和大小
label1.SetBounds(50, 50, 100, 30);
label2.SetBounds(50, 100, 100, 30);
label3.SetBounds(50, 150, 100, 30);
// 将 Label 控件绘制到打印页面上
label1.DrawToBitmap(new Bitmap(label1.Width, label1.Height), new Rectangle(0, 0, label1.Width, label1.Height));
label2.DrawToBitmap(new Bitmap(label2.Width, label2.Height), new Rectangle(0, 0, label2.Width, label2.Height));
label3.DrawToBitmap(new Bitmap(label3.Width, label3.Height), new Rectangle(0, 0, label3.Width, label3.Height));
// 将 Label 控件从打印页面上移除
label1.SetBounds(0, 0, 0, 0);
label2.SetBounds(0, 0, 0, 0);
label3.SetBounds(0, 0, 0, 0);
}
```
在上面的代码中,pd_PrintPage 方法被 PrintDocument 的 PrintPage 事件调用。在该方法中,我们首先设置 Label 控件的位置和大小,然后将它们绘制到一个 Bitmap 对象中,最后将 Label 控件从打印页面上移除。
这样,当你点击打印按钮时,PrintDialog 控件会弹出,当你选择了打印机并点击了“确定”按钮后,Label 控件就会被打印出来。
阅读全文
相关推荐


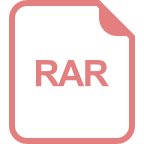
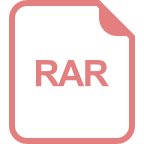
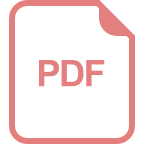
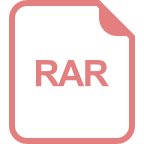
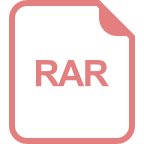
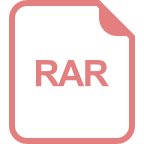
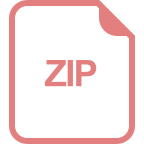
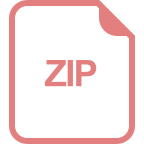
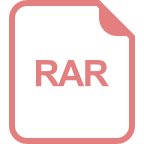
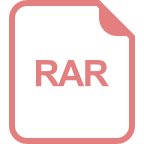
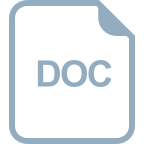
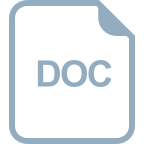
