android上传视频到服务器代码实现
时间: 2023-06-10 12:03:39 浏览: 54
好的,关于android上传视频到服务器的代码实现,以下是一个简单的示例:
1. 在android应用中,首先需要选择一个视频文件并打开它,可以使用Android Intent来实现:
```
Intent intent = new Intent();
intent.setType("video/*");
intent.setAction(Intent.ACTION_GET_CONTENT);
startActivityForResult(Intent.createChooser(intent, "Select a Video "), SELECT_VIDEO);
```
2. 选择完视频文件后,就需要将其上传到服务器了,可以使用HTTPURLConnection来进行上传:
```
public int uploadVideo(String sourceFileUri) {
String upLoadServerUri = "http://example.com/your_php_script.php";
String fileName = sourceFileUri;
HttpURLConnection conn = null;
DataOutputStream dos = null;
String lineEnd = "\r\n";
String twoHyphens = "--";
String boundary = "*****";
int serverResponseCode = 0;
try {
FileInputStream fileInputStream = new FileInputStream(new File(sourceFileUri));
URL url = new URL(upLoadServerUri);
conn = (HttpURLConnection) url.openConnection();
conn.setDoInput(true);
conn.setDoOutput(true);
conn.setUseCaches(false);
conn.setRequestMethod("POST");
conn.setRequestProperty("Connection", "Keep-Alive");
conn.setRequestProperty("ENCTYPE", "multipart/form-data");
conn.setRequestProperty("Content-Type", "multipart/form-data;boundary=" + boundary);
conn.setRequestProperty("uploaded_file", fileName);
dos = new DataOutputStream(conn.getOutputStream());
dos.writeBytes(twoHyphens + boundary + lineEnd);
dos.writeBytes("Content-Disposition: form-data; name=\"uploaded_file\";filename=\""
+ fileName + "\"" + lineEnd);
dos.writeBytes(lineEnd);
byte[] buffer = new byte[1024];
int bytesRead = 0;
while ((bytesRead = fileInputStream.read(buffer)) > 0) {
dos.write(buffer, 0, bytesRead);
}
dos.writeBytes(lineEnd);
dos.writeBytes(twoHyphens + boundary + twoHyphens + lineEnd);
serverResponseCode = conn.getResponseCode();
String serverResponseMessage = conn.getResponseMessage();
fileInputStream.close();
dos.flush();
dos.close();
} catch (Exception e) {
e.printStackTrace();
}
return serverResponseCode;
}
```
这段代码将视频文件通过HTTP POST请求上传到服务器上,需要替换upLoadServerUri为你的服务器地址,同时还需要在你的服务器上编写相应的PHP脚本,来处理上传的视频。
希望这个简单示例能够帮助到你,如果还有什么问题,请随时提出。
相关推荐
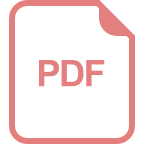
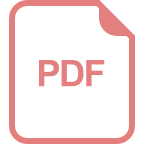
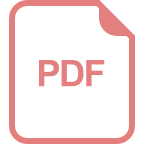














