帮我编写一个Javase的教务管理系统项目,要求有java和jdbc+mysql
时间: 2024-03-18 17:41:49 浏览: 19
好的,下面是一个简单的Javase教务管理系统项目示例,使用了Java和JDBC+MySQL。这个项目包括学生信息管理、课程管理、成绩管理等功能。
首先,您需要在MySQL中创建一个名为"edu_system"的数据库,并创建以下表格:
1. 学生表格(student):
字段名 | 类型 | 约束
id | int | primary key
name | varchar(50) | not null
age | int | not null
sex | varchar(10) | not null
2. 课程表格(course):
字段名 | 类型 | 约束
id | int | primary key
name | varchar(50) | not null
teacher | varchar(50) | not null
3. 成绩表格(score):
字段名 | 类型 | 约束
id | int | primary key
student_id | int | not null
course_id | int | not null
score | int | not null
接下来是Java代码:
1. 数据库连接类(DBUtil.java):
```java
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.SQLException;
public class DBUtil {
private static final String URL = "jdbc:mysql://localhost:3306/edu_system";
private static final String USERNAME = "root";
private static final String PASSWORD = "123456";
public static Connection getConnection() {
Connection conn = null;
try {
Class.forName("com.mysql.jdbc.Driver");
conn = DriverManager.getConnection(URL, USERNAME, PASSWORD);
} catch (ClassNotFoundException | SQLException e) {
e.printStackTrace();
}
return conn;
}
}
```
2. 学生类(Student.java):
```java
public class Student {
private int id;
private String name;
private int age;
private String sex;
// 构造函数、getters和setters省略
// 添加学生
public void add() {
String sql = "INSERT INTO student (name, age, sex) VALUES (?, ?, ?)";
try (Connection conn = DBUtil.getConnection();
PreparedStatement pstmt = conn.prepareStatement(sql)) {
pstmt.setString(1, this.name);
pstmt.setInt(2, this.age);
pstmt.setString(3, this.sex);
pstmt.executeUpdate();
} catch (SQLException e) {
e.printStackTrace();
}
}
// 更新学生信息
public void update() {
String sql = "UPDATE student SET name=?, age=?, sex=? WHERE id=?";
try (Connection conn = DBUtil.getConnection();
PreparedStatement pstmt = conn.prepareStatement(sql)) {
pstmt.setString(1, this.name);
pstmt.setInt(2, this.age);
pstmt.setString(3, this.sex);
pstmt.setInt(4, this.id);
pstmt.executeUpdate();
} catch (SQLException e) {
e.printStackTrace();
}
}
// 删除学生
public void delete() {
String sql = "DELETE FROM student WHERE id=?";
try (Connection conn = DBUtil.getConnection();
PreparedStatement pstmt = conn.prepareStatement(sql)) {
pstmt.setInt(1, this.id);
pstmt.executeUpdate();
} catch (SQLException e) {
e.printStackTrace();
}
}
// 查询学生信息
public static List<Student> query() {
List<Student> students = new ArrayList<>();
String sql = "SELECT * FROM student";
try (Connection conn = DBUtil.getConnection();
PreparedStatement pstmt = conn.prepareStatement(sql);
ResultSet rs = pstmt.executeQuery()) {
while (rs.next()) {
Student student = new Student();
student.setId(rs.getInt("id"));
student.setName(rs.getString("name"));
student.setAge(rs.getInt("age"));
student.setSex(rs.getString("sex"));
students.add(student);
}
} catch (SQLException e) {
e.printStackTrace();
}
return students;
}
}
```
3. 课程类(Course.java):
```java
public class Course {
private int id;
private String name;
private String teacher;
// 构造函数、getters和setters省略
// 添加课程
public void add() {
String sql = "INSERT INTO course (name, teacher) VALUES (?, ?)";
try (Connection conn = DBUtil.getConnection();
PreparedStatement pstmt = conn.prepareStatement(sql)) {
pstmt.setString(1, this.name);
pstmt.setString(2, this.teacher);
pstmt.executeUpdate();
} catch (SQLException e) {
e.printStackTrace();
}
}
// 更新课程信息
public void update() {
String sql = "UPDATE course SET name=?, teacher=? WHERE id=?";
try (Connection conn = DBUtil.getConnection();
PreparedStatement pstmt = conn.prepareStatement(sql)) {
pstmt.setString(1, this.name);
pstmt.setString(2, this.teacher);
pstmt.setInt(3, this.id);
pstmt.executeUpdate();
} catch (SQLException e) {
e.printStackTrace();
}
}
// 删除课程
public void delete() {
String sql = "DELETE FROM course WHERE id=?";
try (Connection conn = DBUtil.getConnection();
PreparedStatement pstmt = conn.prepareStatement(sql)) {
pstmt.setInt(1, this.id);
pstmt.executeUpdate();
} catch (SQLException e) {
e.printStackTrace();
}
}
// 查询课程信息
public static List<Course> query() {
List<Course> courses = new ArrayList<>();
String sql = "SELECT * FROM course";
try (Connection conn = DBUtil.getConnection();
PreparedStatement pstmt = conn.prepareStatement(sql);
ResultSet rs = pstmt.executeQuery()) {
while (rs.next()) {
Course course = new Course();
course.setId(rs.getInt("id"));
course.setName(rs.getString("name"));
course.setTeacher(rs.getString("teacher"));
courses.add(course);
}
} catch (SQLException e) {
e.printStackTrace();
}
return courses;
}
}
```
4. 成绩类(Score.java):
```java
public class Score {
private int id;
private int studentId;
private int courseId;
private int score;
// 构造函数、getters和setters省略
// 添加成绩
public void add() {
String sql = "INSERT INTO score (student_id, course_id, score) VALUES (?, ?, ?)";
try (Connection conn = DBUtil.getConnection();
PreparedStatement pstmt = conn.prepareStatement(sql)) {
pstmt.setInt(1, this.studentId);
pstmt.setInt(2, this.courseId);
pstmt.setInt(3, this.score);
pstmt.executeUpdate();
} catch (SQLException e) {
e.printStackTrace();
}
}
// 更新成绩
public void update() {
String sql = "UPDATE score SET score=? WHERE id=?";
try (Connection conn = DBUtil.getConnection();
PreparedStatement pstmt = conn.prepareStatement(sql)) {
pstmt.setInt(1, this.score);
pstmt.setInt(2, this.id);
pstmt.executeUpdate();
} catch (SQLException e) {
e.printStackTrace();
}
}
// 删除成绩
public void delete() {
String sql = "DELETE FROM score WHERE id=?";
try (Connection conn = DBUtil.getConnection();
PreparedStatement pstmt = conn.prepareStatement(sql)) {
pstmt.setInt(1, this.id);
pstmt.executeUpdate();
} catch (SQLException e) {
e.printStackTrace();
}
}
// 查询成绩信息
public static List<Score> query() {
List<Score> scores = new ArrayList<>();
String sql = "SELECT * FROM score";
try (Connection conn = DBUtil.getConnection();
PreparedStatement pstmt = conn.prepareStatement(sql);
ResultSet rs = pstmt.executeQuery()) {
while (rs.next()) {
Score score = new Score();
score.setId(rs.getInt("id"));
score.setStudentId(rs.getInt("student_id"));
score.setCourseId(rs.getInt("course_id"));
score.setScore(rs.getInt("score"));
scores.add(score);
}
} catch (SQLException e) {
e.printStackTrace();
}
return scores;
}
}
```
以上是一个简单的JavaSE教务管理系统项目,包括学生信息管理、课程管理、成绩管理等功能,使用了Java和JDBC+MySQL。
相关推荐
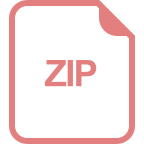














