java 移除注解
时间: 2023-06-21 11:17:35 浏览: 164
要移除Java代码中的注解,可以使用反射API中的Annotation类的方法来实现。具体步骤如下:
1. 获取需要移除注解的类或方法等元素的Class对象;
2. 使用Class对象的getDeclaredAnnotations()方法获取所有的注解对象;
3. 遍历注解对象数组,判断需要移除的注解类型,如果匹配,则通过反射API中的AnnotatedElement接口的方法来移除注解。
示例代码如下:
```java
import java.lang.annotation.Annotation;
import java.lang.reflect.AnnotatedElement;
public class AnnotationUtils {
/**
* 移除指定类型的注解
*
* @param element 需要移除注解的元素
* @param clazz 需要移除的注解类型
*/
public static void removeAnnotation(AnnotatedElement element, Class<? extends Annotation> clazz) {
Annotation[] annotations = element.getDeclaredAnnotations();
for (Annotation annotation : annotations) {
if (annotation.annotationType().equals(clazz)) {
try {
// 使用反射API中的方法移除注解
Class<?> annotationType = annotation.annotationType();
Method method = annotationType.getDeclaredMethod("value");
method.setAccessible(true);
Object value = method.invoke(annotation);
InvocationHandler handler = (proxy, method1, args) -> value;
Annotation proxyAnnotation = (Annotation) Proxy.newProxyInstance(annotationType.getClassLoader(),
new Class[]{annotationType}, handler);
Method setMethod = element.getClass().getMethod("set" + annotationType.getSimpleName(), annotationType);
setMethod.invoke(element, proxyAnnotation);
} catch (Exception e) {
throw new RuntimeException("移除注解失败", e);
}
}
}
}
}
```
使用示例:
```java
@MyAnnotation("test")
public class Test {
@MyAnnotation("test")
public void test() {
}
}
// 移除类注解
Class<Test> clazz = Test.class;
AnnotationUtils.removeAnnotation(clazz, MyAnnotation.class);
// 移除方法注解
Method method = clazz.getMethod("test");
AnnotationUtils.removeAnnotation(method, MyAnnotation.class);
```
阅读全文
相关推荐









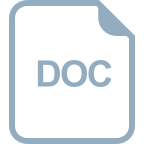





