使用html5 canvas 做一个可以渲染1000条JSON数据的表格
时间: 2024-03-16 19:45:14 浏览: 13
好的,我们可以使用 Canvas 绘制一个简单的表格来展示 JSON 数据。以下是示例代码:
HTML 文件:
```
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Canvas Example</title>
</head>
<body>
<canvas id="myCanvas"></canvas>
<script src="script.js"></script>
</body>
</html>
```
JavaScript 文件:
```
// 获取 Canvas 元素
var canvas = document.getElementById("myCanvas");
// 获取 Canvas 上下文
var ctx = canvas.getContext("2d");
// 定义 JSON 数据
var data = [
{name: "Alice", age: 20, score: 80},
{name: "Bob", age: 22, score: 90},
// ... 1000 条数据
];
// 定义表格参数
var table = {
x: 10,
y: 10,
width: 500,
height: 500,
columns: ["Name", "Age", "Score"],
columnWidths: [200, 100, 100],
rowHeight: 30,
headerColor: "#ccc",
rowColor1: "#f0f0f0",
rowColor2: "#ffffff"
};
// 渲染函数
function render() {
// 清空画布
ctx.clearRect(0, 0, canvas.width, canvas.height);
// 绘制表格
ctx.fillStyle = table.headerColor;
ctx.fillRect(table.x, table.y, table.width, table.rowHeight);
ctx.fillStyle = "#000000";
for (var i = 0; i < table.columns.length; i++) {
ctx.fillText(table.columns[i], table.x + 10 + i * table.columnWidths[i], table.y + table.rowHeight / 2 + 5);
}
var rowY = table.y + table.rowHeight;
for (var i = 0; i < data.length; i++) {
ctx.fillStyle = i % 2 == 0 ? table.rowColor1 : table.rowColor2;
ctx.fillRect(table.x, rowY, table.width, table.rowHeight);
ctx.fillStyle = "#000000";
ctx.fillText(data[i].name, table.x + 10, rowY + table.rowHeight / 2 + 5);
ctx.fillText(data[i].age, table.x + 10 + table.columnWidths[0], rowY + table.rowHeight / 2 + 5);
ctx.fillText(data[i].score, table.x + 10 + table.columnWidths[0] + table.columnWidths[1], rowY + table.rowHeight / 2 + 5);
rowY += table.rowHeight;
}
}
// 调用渲染函数
render();
```
以上代码会在 Canvas 上绘制一个包含表头和 1000 行数据的表格,可以根据需要自行修改。注意,在实际应用中,应该使用异步加载数据,以避免页面卡顿。
相关推荐
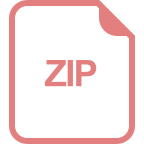
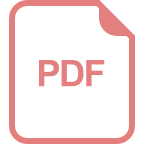














