joinShopCar() { alert("加入购物车成功"); let shopcarList = JSON.parse(localStorage.getItem("shopcar")) || []; // console.log(shopcarList); this.goodsData.num = 1; this.goodsData.isChecked = false; let ind = shopcarList.findIndex((ele) => { return ele.id == this.goodsId; }); // console.log(ind); // 如果有相同的返回该数据的下标,没有则返回-1 if (ind != -1) { shopcarList[ind].num++; } else { shopcarList.push(this.goodsData); } localStorage.setItem("shopcar", JSON.stringify(shopcarList)); this.$router.push("/shopcar"); },什么意思
时间: 2024-03-01 16:49:15 浏览: 315
这是一个Vue.js方法,用于将当前商品加入购物车。方法的具体实现如下:
首先,弹出一个提示框,提示用户“加入购物车成功”。
然后,从本地存储中获取名为“shopcar”的数据,如果没有则初始化一个空数组。
接着,将当前商品的数量设置为1,并将其选中状态设置为false。
接下来,查找当前商品在“shopcar”中的下标,如果存在则将该商品数量加1,如果不存在则将当前商品添加到“shopcar”中。
最后,将“shopcar”数据重新存储到本地存储中,并跳转到购物车页面。
总之,这个方法的主要作用是将当前商品添加到购物车,并更新购物车数据。
相关问题
// 加入购物车 joinShopCar() { alert("加入购物车成功"); let shopcarList = JSON.parse(localStorage.getItem("shopcar")) || []; console.log(shopcarList); this.goodsData.num = 1; this.goodsData.isChecked = false; let ind = shopcarList.findIndex((ele) => { return ele.id == this.goodsId.id; }); console.log(ind); // 如果有相同的返回该数据的下标,没有则返回-1 if (ind == -1) { shopcarList.push(this.goodsData); } else { shopcarList[ind].num++; } localStorage.setItem("shopcar", JSON.stringify(shopcarList)); this.$router.push("/shopcar"); },
这段代码是关于一个加入购物车的函数,当用户点击“加入购物车”按钮时,会向用户弹出一个提示框,然后会从本地存储中获取购物车列表(如果没有则初始化为空数组),并将当前商品的数量设置为1,选中状态设置为false。接着会使用findIndex方法查找购物车列表中是否有与当前商品相同的商品,如果有则返回该商品的下标,否则返回-1。如果返回的下标是-1,则表示购物车列表中没有相同的商品,此时将当前商品数据加入购物车列表中,否则将该商品的数量加1。最后将更新后的购物车列表重新存储到本地存储中,并跳转到购物车页面。
这段代码怎么优化export default { data() { return { stepper: 1, shopcarList: [], }; }, methods: { onSubmit() { console.log(111); }, add(index) { // console.log(index); this.$set( this.shopcarList[index], this.shopcarList[index].num, this.shopcarList[index].num++ ); }, del(index) { if (this.shopcarList[index].num == 1) { this.$toast("不能再减了"); } else { this.$set( this.shopcarList[index], this.shopcarList[index].num, this.shopcarList[index].num-- ); } }, }, created() { this.shopcarList = JSON.parse(localStorage.getItem("shopcar")) || []; console.log(this.shopcarList); }, computed: { isAllChecked: { set(val) { this.shopcarList.forEach((ele) => { ele.isChecked = val; }); }, get() { return this.shopcarList.every((ele) => { return ele.isChecked == true; }); }, }, priceTotal() { let sum = 0; let newPrice = this.shopcarList.filter((ele) => { return ele.isChecked == true; }); sum = newPrice.reduce((pre, current) => { return (pre += current.price * current.num); }, 0); return sum * 100; }, }, }; </script>
这段代码可以进行以下优化:
1. 将 `methods` 中的 `add` 和 `del` 方法中的 `$set` 改为直接修改属性值,例如 `this.shopcarList[index].num++` 和 `this.shopcarList[index].num--`。
2. 在 `computed` 中的 `priceTotal` 方法中,可以使用 `filter` 和 `reduce` 方法的链式调用,将其简化为一行代码。
优化后的代码如下:
```
export default {
data() {
return {
stepper: 1,
shopcarList: [],
};
},
methods: {
onSubmit() {
console.log(111);
},
add(index) {
this.shopcarList[index].num++;
},
del(index) {
if (this.shopcarList[index].num == 1) {
this.$toast("不能再减了");
} else {
this.shopcarList[index].num--;
}
},
},
created() {
this.shopcarList = JSON.parse(localStorage.getItem("shopcar")) || [];
console.log(this.shopcarList);
},
computed: {
isAllChecked: {
set(val) {
this.shopcarList.forEach((ele) => {
ele.isChecked = val;
});
},
get() {
return this.shopcarList.every((ele) => ele.isChecked);
},
},
priceTotal() {
return this.shopcarList.filter((ele) => ele.isChecked)
.reduce((pre, current) => pre += current.price * current.num, 0) * 100;
},
},
};
```
阅读全文
相关推荐
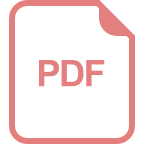
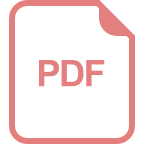
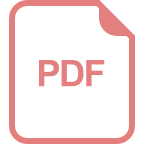





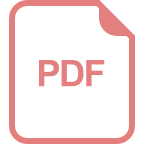




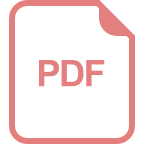
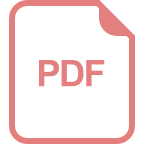