使用Springmvc+jsp,模拟数据(商品数据),当执行springmvc标记的控制器的类的时候,无参构造方法中模拟list集合数据, 将list集合存储起来,完成查询所有,添加商品,删除,和修改商品
时间: 2024-05-09 21:16:41 浏览: 9
首先,我们需要在Spring配置文件中配置SpringMVC的组件扫描、视图解析器和控制器。假设我们的控制器类为`ProductController`,则配置如下:
```xml
<context:component-scan base-package="com.example.controller" />
<mvc:view-resolvers>
<mvc:jsp prefix="/WEB-INF/views/" suffix=".jsp" />
</mvc:view-resolvers>
<mvc:annotation-driven />
```
接下来,我们来编写`ProductController`类。在这个类中,我们需要定义一个`List<Product>`类型的属性,以及对应的增删改查方法。
```java
@Controller
@RequestMapping("/product")
public class ProductController {
private List<Product> productList;
public ProductController() {
// 模拟商品数据
productList = new ArrayList<>();
productList.add(new Product(1, "商品1", 100.0));
productList.add(new Product(2, "商品2", 200.0));
productList.add(new Product(3, "商品3", 300.0));
}
@RequestMapping("/list")
public ModelAndView list() {
ModelAndView modelAndView = new ModelAndView("product/list");
modelAndView.addObject("productList", productList);
return modelAndView;
}
@RequestMapping("/add")
public String add(Product product) {
productList.add(product);
return "redirect:/product/list";
}
@RequestMapping("/delete")
public String delete(int id) {
productList.removeIf(product -> product.getId() == id);
return "redirect:/product/list";
}
@RequestMapping("/edit")
public ModelAndView edit(int id) {
ModelAndView modelAndView = new ModelAndView("product/edit");
modelAndView.addObject("product", getProductById(id));
return modelAndView;
}
@RequestMapping("/update")
public String update(Product product) {
Product oldProduct = getProductById(product.getId());
oldProduct.setName(product.getName());
oldProduct.setPrice(product.getPrice());
return "redirect:/product/list";
}
private Product getProductById(int id) {
for (Product product : productList) {
if (product.getId() == id) {
return product;
}
}
return null;
}
}
```
在这个类中,我们首先在无参构造方法中模拟了一些商品数据,然后定义了对应的增删改查方法。
`list`方法用于查询所有商品,并将结果存入ModelAndView对象中返回;`add`方法用于添加商品,将新商品添加到商品列表中,并重定向到商品列表页面;`delete`方法用于删除商品,通过商品ID查找到对应的商品并将其从商品列表中删除,并重定向到商品列表页面;`edit`方法用于编辑商品,通过商品ID查找到对应的商品并将其存入ModelAndView对象中返回;`update`方法用于更新商品,通过商品ID查找到对应的商品并将其更新为新商品的信息,并重定向到商品列表页面。其中,`@RequestMapping`注解用于指定每个方法对应的请求路径。
最后,我们需要在`/WEB-INF/views`目录下创建对应的JSP页面,如`list.jsp`、`add.jsp`、`edit.jsp`等,用于展示商品列表、添加商品、编辑商品等操作的页面。
这样,我们就完成了使用SpringMVC模拟商品数据的任务。
相关推荐
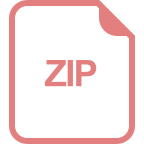














