用c++写一个词法分析程序
时间: 2023-07-01 22:13:04 浏览: 91
好的,下面是一个简单的词法分析器的示例代码,使用C++语言实现:
```c++
#include <iostream>
#include <string>
#include <vector>
using namespace std;
// Token结构体,用于存储词法分析结果
struct Token {
string type; // Token类型
string value; // Token值
};
// 词法分析函数,参数为待分析的代码字符串
vector<Token> lexer(string code) {
vector<Token> tokens; // 存储Token的vector
string current_token = ""; // 当前Token的值
string current_type = ""; // 当前Token的类型
// 遍历代码字符串,进行词法分析
for (int i = 0; i < code.size(); i++) {
char ch = code[i];
// 判断是否为数字
if (isdigit(ch)) {
current_token += ch;
current_type = "NUMBER";
}
// 判断是否为字母
else if (isalpha(ch)) {
current_token += ch;
current_type = "IDENTIFIER";
}
// 判断是否为操作符
else if (ch == '+' || ch == '-' || ch == '*' || ch == '/') {
// 如果当前Token不为空,则将其加入tokens中
if (current_token != "") {
tokens.push_back({current_type, current_token});
current_token = "";
current_type = "";
}
// 将操作符作为一个Token加入tokens中
tokens.push_back({"OPERATOR", string(1, ch)});
}
// 判断是否为分隔符
else if (ch == '(' || ch == ')' || ch == '{' || ch == '}') {
// 如果当前Token不为空,则将其加入tokens中
if (current_token != "") {
tokens.push_back({current_type, current_token});
current_token = "";
current_type = "";
}
// 将分隔符作为一个Token加入tokens中
tokens.push_back({"SEPARATOR", string(1, ch)});
}
// 判断是否为空格
else if (isspace(ch)) {
// 如果当前Token不为空,则将其加入tokens中
if (current_token != "") {
tokens.push_back({current_type, current_token});
current_token = "";
current_type = "";
}
}
}
// 将最后一个Token加入tokens中
if (current_token != "") {
tokens.push_back({current_type, current_token});
}
return tokens;
}
// 测试函数
int main() {
string code = "int sum(int a, int b) { return a + b; }";
vector<Token> tokens = lexer(code);
for (int i = 0; i < tokens.size(); i++) {
cout << tokens[i].type << ": " << tokens[i].value << endl;
}
return 0;
}
```
该程序接收一个字符串代码作为输入,返回一个Token的vector,其中每个Token包含一个类型和一个值。该程序可以识别数字、标识符、操作符和分隔符,并将其作为Token返回。
阅读全文
相关推荐





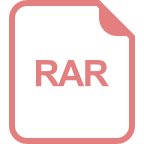





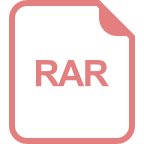

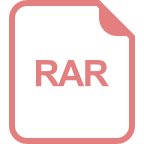
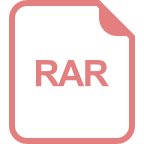
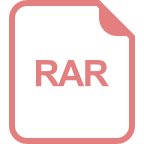

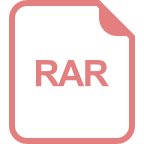