python网上投票系统
时间: 2023-06-22 19:43:02 浏览: 53
好的,您需要了解如何构建一个 Python 网上投票系统。首先,您可以使用 Django 框架来构建您的应用程序。这个框架是一个流行的 Python Web 框架,它提供了许多有用的功能,例如 ORM(对象关系映射)、表单、认证和安全性等。
在 Django 中,您可以创建一个名为 Polls 的应用程序,这个应用程序将包含所有与投票相关的功能。您可以创建一个模型来表示您的投票,例如:
```
from django.db import models
class Poll(models.Model):
question = models.CharField(max_length=200)
pub_date = models.DateTimeField('date published')
class Choice(models.Model):
poll = models.ForeignKey(Poll, on_delete=models.CASCADE)
choice_text = models.CharField(max_length=200)
votes = models.IntegerField(default=0)
```
这个模型包含了一个问题和一个发布日期,以及一个选项和一个投票数。接下来,您可以创建一个视图来处理投票:
```
from django.shortcuts import get_object_or_404, render
from django.http import HttpResponseRedirect
from django.urls import reverse
from .models import Choice, Poll
def vote(request, poll_id):
poll = get_object_or_404(Poll, pk=poll_id)
try:
selected_choice = poll.choice_set.get(pk=request.POST['choice'])
except (KeyError, Choice.DoesNotExist):
return render(request, 'polls/detail.html', {
'poll': poll,
'error_message': "You didn't select a choice.",
})
else:
selected_choice.votes += 1
selected_choice.save()
return HttpResponseRedirect(reverse('polls:results', args=(poll.id,)))
```
这个视图将获取投票 ID,然后查找对应的投票。如果用户没有选择选项,则会显示错误消息。如果用户选择了选项,则会将投票数加 1,并将用户重定向到结果页面。
最后,您可以创建一个模板来显示投票的问题和选项:
```
{% extends "base.html" %}
{% block content %}
<h1>{{ poll.question }}</h1>
{% if error_message %}
<p><strong>{{ error_message }}</strong></p>
{% endif %}
<form action="{% url 'polls:vote' poll.id %}" method="post">
{% csrf_token %}
{% for choice in poll.choice_set.all %}
<input type="radio" name="choice" id="choice{{ forloop.counter }}" value="{{ choice.id }}">
<label for="choice{{ forloop.counter }}">{{ choice.choice_text }}</label><br>
{% endfor %}
<input type="submit" value="Vote">
</form>
{% endblock %}
```
这个模板包含了投票的问题和选项,并允许用户选择选项并提交表单。
这是一个基本的 Python 网上投票系统的实现,您可以根据自己的需求进行修改和扩展。
相关推荐
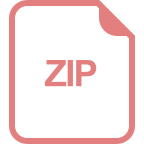
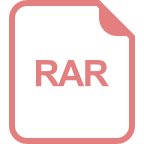
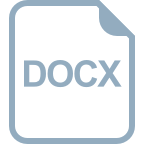
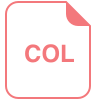













