利用JavaScript写一个简易的打地鼠游戏,要求页面美观,可以随机刷新地鼠位置,打到地鼠获取积分等
时间: 2024-03-26 11:38:35 浏览: 45
好的,以下是一个简易的打地鼠游戏的代码实现:
HTML代码:
```html
<!DOCTYPE html>
<html>
<head>
<title>打地鼠</title>
<meta charset="UTF-8">
<style>
body {
text-align: center;
padding-top: 50px;
background-color: #f3f3f3;
font-family: Arial, sans-serif;
}
h1 {
color: #333;
}
#game {
display: inline-block;
position: relative;
}
.hole {
display: inline-block;
margin: 5px;
position: relative;
width: 100px;
height: 100px;
}
.hole img {
position: absolute;
top: 0;
left: 0;
display: none;
width: 100px;
height: 100px;
}
.score {
font-size: 24px;
margin-top: 50px;
}
</style>
</head>
<body>
<h1>打地鼠</h1>
<div id="game">
<div class="hole" id="hole1"><img src="hole.png"><img src="mole.png"></div>
<div class="hole" id="hole2"><img src="hole.png"><img src="mole.png"></div>
<div class="hole" id="hole3"><img src="hole.png"><img src="mole.png"></div>
<div class="hole" id="hole4"><img src="hole.png"><img src="mole.png"></div>
<div class="hole" id="hole5"><img src="hole.png"><img src="mole.png"></div>
<div class="hole" id="hole6"><img src="hole.png"><img src="mole.png"></div>
<div class="hole" id="hole7"><img src="hole.png"><img src="mole.png"></div>
<div class="hole" id="hole8"><img src="hole.png"><img src="mole.png"></div>
<div class="hole" id="hole9"><img src="hole.png"><img src="mole.png"></div>
</div>
<div class="score">得分:<span id="score">0</span></div>
<script src="game.js"></script>
</body>
</html>
```
JavaScript代码:
```javascript
// 获取元素
var holes = document.querySelectorAll('.hole');
var scoreBoard = document.getElementById('score');
// 初始化变量
var lastHole;
var timeUp = false;
var score = 0;
// 随机时间函数
function randomTime(min, max) {
return Math.round(Math.random() * (max - min) + min);
}
// 随机地洞函数
function randomHole(holes) {
var idx = Math.floor(Math.random() * holes.length);
var hole = holes[idx];
if (hole === lastHole) {
return randomHole(holes);
}
lastHole = hole;
return hole;
}
// 出现地鼠函数
function peep() {
var time = randomTime(200, 1000);
var hole = randomHole(holes);
hole.classList.add('up');
setTimeout(function() {
hole.classList.remove('up');
if (!timeUp) peep();
}, time);
}
// 开始游戏函数
function startGame() {
scoreBoard.textContent = 0;
timeUp = false;
score = 0;
peep();
setTimeout(function() {
timeUp = true;
}, 10000);
}
// 击打地鼠函数
function bonk(e) {
if (!e.isTrusted) return;
score++;
this.parentNode.classList.remove('up');
scoreBoard.textContent = score;
}
// 监听击打事件
holes.forEach(function(hole) {
hole.addEventListener('click', bonk);
});
```
CSS代码:
```css
.up .mole {
transform: translateY(0);
}
```
同样地,本代码只是一个简单的示例,还有很多可以改进的地方,例如增加音效、增加难度、增加排行榜等等。
阅读全文
相关推荐
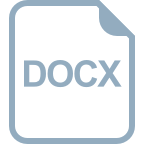
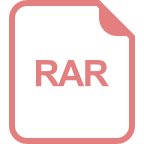
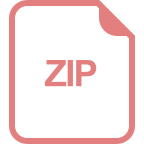
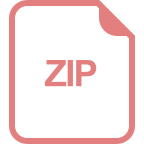
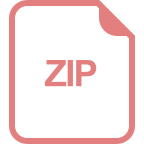
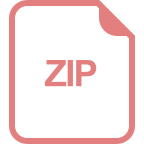
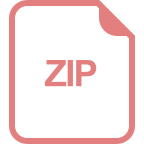
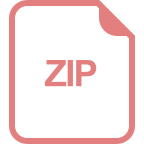
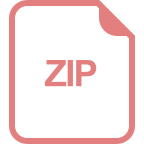
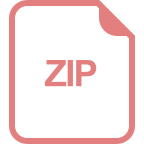
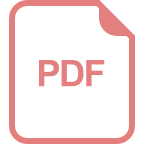
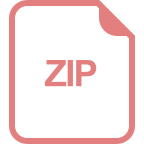
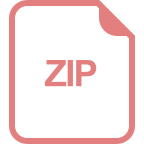
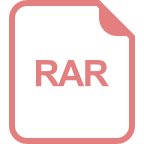
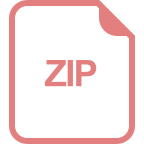
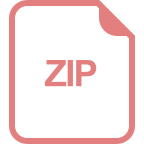
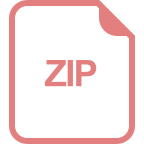