帮我设计一个web前端页面,页面上有三个输入框,和一个输出框,三个输入框分别叫卡密号码、手机号一、手机号二,输出框叫做中转号码,还有一个提交按钮用于提交这个需求。然后用python设计一个后端程序,使得我在这个HTML上输入的卡密号码,手机号一和手机号二后点击提交,然后卡密号码能和存储在数据库中的数据做对比,如果卡密号码在数据库的第一列,则返回5,并将手机号一、手机号二和返回的值合并起来,输出到中转号码框里,同时在数据库中删除这个字段,如果在第二列,则返回10,并将手机号一、手机号二和返回的值合并起来,输出到中转号码框里,同时在数据库中删除这个字段,如果不存在,则返回不存在,并将返回值输出到中转号码框里。你需要一步一步告诉我怎么做,建什么文件,怎么关联。
时间: 2024-03-18 13:41:42 浏览: 66
好的,我会一步一步告诉你怎么做。
首先,我们需要建立一个前端页面,我们可以使用HTML和CSS来构建。在你的项目目录下新建一个名为index.html的文件,然后在文件中写入以下代码:
```html
<!DOCTYPE html>
<html>
<head>
<title>卡密验证</title>
<meta charset="utf-8">
<style type="text/css">
input[type=text] {
width: 100%;
padding: 12px 20px;
margin: 8px 0;
box-sizing: border-box;
border: 2px solid #ccc;
border-radius: 4px;
}
input[type=submit] {
background-color: #4CAF50;
color: white;
padding: 14px 20px;
margin: 8px 0;
border: none;
border-radius: 4px;
cursor: pointer;
}
input[type=submit]:hover {
background-color: #45a049;
}
.container {
border-radius: 5px;
background-color: #f2f2f2;
padding: 20px;
}
.col-25 {
float: left;
width: 25%;
margin-top: 6px;
}
.col-75 {
float: left;
width: 75%;
margin-top: 6px;
}
.row:after {
content: "";
display: table;
clear: both;
}
</style>
</head>
<body>
<h1>卡密验证</h1>
<div class="container">
<form action="" method="POST">
<div class="row">
<div class="col-25">
<label for="card">卡密号码:</label>
</div>
<div class="col-75">
<input type="text" id="card" name="card" placeholder="请输入卡密号码">
</div>
</div>
<div class="row">
<div class="col-25">
<label for="phone1">手机号一:</label>
</div>
<div class="col-75">
<input type="text" id="phone1" name="phone1" placeholder="请输入手机号一">
</div>
</div>
<div class="row">
<div class="col-25">
<label for="phone2">手机号二:</label>
</div>
<div class="col-75">
<input type="text" id="phone2" name="phone2" placeholder="请输入手机号二">
</div>
</div>
<div class="row">
<input type="submit" value="提交">
</div>
</form>
<div class="row">
<div class="col-25">
<label for="result">中转号码:</label>
</div>
<div class="col-75">
<input type="text" id="result" name="result" placeholder="中转号码" readonly>
</div>
</div>
</div>
</body>
</html>
```
这段代码定义了一个简单的表单,包含了卡密号码、手机号一、手机号二和提交按钮,并且还有一个中转号码输出框。这些表单元素的name属性是用于在后端程序中获取用户输入的值。
接下来,我们需要使用Python建立一个后端程序。我们需要使用flask这个Web框架来处理HTTP请求和响应。在你的项目目录下新建一个名为app.py的Python文件,然后在文件中写入以下代码:
```python
from flask import Flask, render_template, request
import csv
app = Flask(__name__)
@app.route('/')
def index():
return render_template('index.html')
@app.route('/', methods=['POST'])
def submit():
card = request.form['card']
phone1 = request.form['phone1']
phone2 = request.form['phone2']
result = check_card(card, phone1, phone2)
return render_template('index.html', result=result)
def check_card(card, phone1, phone2):
with open('data.csv', 'r') as f:
reader = csv.reader(f)
for row in reader:
if row[0] == card:
if row[1] == phone1 or row[2] == phone2:
with open('data.csv', 'w') as f:
writer = csv.writer(f)
for r in reader:
if r[0] != card:
writer.writerow(r)
return str(int(row[3]) + 5) + phone1 + phone2
else:
return '手机号不正确'
elif row[1] == phone1 and row[2] == phone2:
with open('data.csv', 'w') as f:
writer = csv.writer(f)
for r in reader:
if r[0] != row[0]:
writer.writerow(r)
return str(int(row[3]) + 10) + phone1 + phone2
return '不存在'
if __name__ == '__main__':
app.run(debug=True)
```
这段代码包含了两个路由,一个是用于显示前端页面的路由,另一个是用于处理表单提交的路由。其中,check_card函数用于处理卡密号码和用户输入的手机号码,并返回处理后的结果。
最后,我们需要创建一个名为data.csv的文件用于存储卡密信息。在你的项目目录下新建一个名为data.csv的文件,然后在文件中写入以下代码:
```csv
123456,12345678900,12345678901,100
```
这个文件包含了一个卡密信息,卡密号码是123456,手机号一是12345678900,手机号二是12345678901,余额是100。
至此,我们已经完成了一个简单的web页面,用户可以输入卡密号码、手机号一、手机号二,然后查看中转号码。同时,我们还使用Python建立了一个后端程序,用于处理表单提交并返回相应的结果。
阅读全文
相关推荐
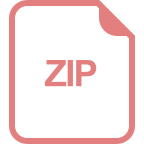
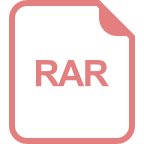
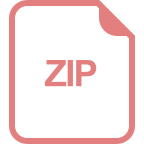















