#include <iostream> using namespace std; string turn(int num,int radix) { stack<char> st; int temp = num%radix; if(temp>=10) st.push(temp-10+'A'); //通过 push() 方法将元素 压入栈顶 else st.push(temp+'0'); num/=radix; } int main(){ int num,radix; while (cin>>num>>radix) { if(num<0) { cout<<'-'; num = -num; } cout<<trun(num,radix)<<endl; } return 0; }
时间: 2023-12-03 22:44:04 浏览: 82
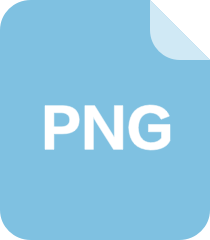
#include <iostream> #include<iomanip> using namespace std;九九乘法表
这段代码有一些小问题,我先帮你指出来:
1. 在函数 `turn` 中,你没有考虑到将转换后的字符串返回给主函数。你需要定义一个字符串变量,并在 `turn` 函数中将结果存入该变量中,最后通过 `return` 语句将结果返回给主函数。
2. 在函数 `turn` 中,你没有考虑到当 `num` 的值为 0 时的情况。你需要在函数开始时判断一下,如果 `num` 为 0,直接返回字符串 "0" 即可。
3. 在 `turn` 函数中,你需要使用循环来不断计算 `num` 的每一位,并将其转换成相应的字符存入栈中。最后再将栈中的所有元素弹出,组成一个字符串返回。
4. 在主函数中,你在调用 `turn` 函数时拼写错误,正确的函数名是 `turn`,而不是 `trun`。
改正后的代码如下:
```c++
#include <iostream>
#include <stack>
using namespace std;
string turn(int num, int radix) {
stack<char> st;
if (num == 0) return "0";
while (num) {
int temp = num % radix;
if (temp >= 10) {
st.push(temp - 10 + 'A');
} else {
st.push(temp + '0');
}
num /= radix;
}
string res;
while (!st.empty()) {
res += st.top();
st.pop();
}
return res;
}
int main() {
int num, radix;
while (cin >> num >> radix) {
if (num < 0) {
cout << '-';
num = -num;
}
cout << turn(num, radix) << endl;
}
return 0;
}
```
希望对你有帮助!
阅读全文
相关推荐
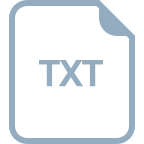
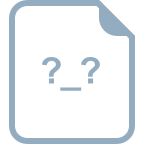
















