java数据库学生信息表
时间: 2023-10-14 20:23:02 浏览: 80
以下是一个简单的学生信息表的Java代码,使用MySQL数据库。
```java
import java.sql.*;
public class StudentInfo {
static final String JDBC_DRIVER = "com.mysql.jdbc.Driver";
static final String DB_URL = "jdbc:mysql://localhost/STUDENT";
static final String USER = "username";
static final String PASS = "password";
public static void main(String[] args) {
Connection conn = null;
Statement stmt = null;
try{
Class.forName("com.mysql.jdbc.Driver");
System.out.println("Connecting to database...");
conn = DriverManager.getConnection(DB_URL,USER,PASS);
System.out.println("Creating statement...");
stmt = conn.createStatement();
String sql;
sql = "SELECT id, name, age, gender, major FROM student_info";
ResultSet rs = stmt.executeQuery(sql);
while(rs.next()){
int id = rs.getInt("id");
String name = rs.getString("name");
int age = rs.getInt("age");
String gender = rs.getString("gender");
String major = rs.getString("major");
System.out.print("ID: " + id);
System.out.print(", Name: " + name);
System.out.print(", Age: " + age);
System.out.print(", Gender: " + gender);
System.out.println(", Major: " + major);
}
rs.close();
stmt.close();
conn.close();
} catch(SQLException se) {
se.printStackTrace();
} catch(Exception e) {
e.printStackTrace();
} finally {
try{
if(stmt!=null)
stmt.close();
} catch(SQLException se2) {
}
try {
if(conn!=null)
conn.close();
} catch(SQLException se) {
se.printStackTrace();
}
}
System.out.println("Goodbye!");
}
}
```
在此示例中,我们连接到名为“STUDENT”的数据库,并从名为“student_info”的表中检索学生信息。数据从结果集中抓取并打印到控制台。您需要将“username”和“password”替换为您的MySQL数据库的实际用户名和密码,以便此示例正确运行。
阅读全文
相关推荐
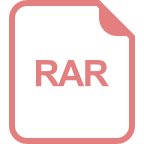
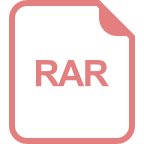
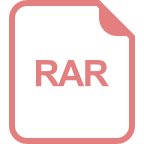
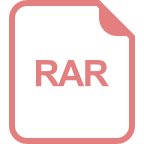
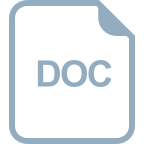
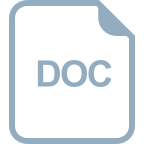
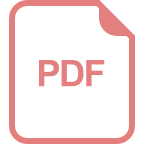
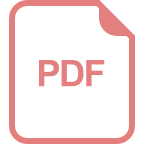
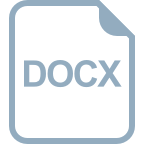
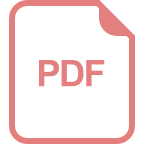
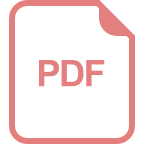
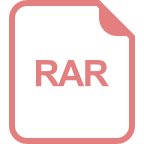
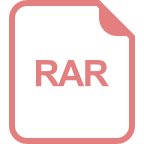
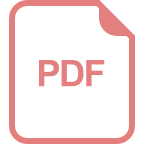
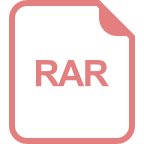