如何用近似算法求解顶点覆盖问题c或c++
时间: 2024-02-24 17:58:34 浏览: 20
以下是使用C++实现的贪心算法求解顶点覆盖问题的代码:
```c++
#include <iostream>
#include <vector>
#include <set>
using namespace std;
// 顶点覆盖问题的贪心算法
set<int> vertexCover(vector<pair<int, int>>& edges) {
set<int> cover; // 存放顶点覆盖集合
while (!edges.empty()) {
int u = edges.back().first;
int v = edges.back().second;
edges.pop_back();
cover.insert(u);
cover.insert(v);
for (auto it = edges.begin(); it != edges.end();) {
if (it->first == u || it->second == u ||
it->first == v || it->second == v) {
it = edges.erase(it);
} else {
++it;
}
}
}
return cover;
}
// 测试
int main() {
// 构造一个无向图
vector<pair<int, int>> edges = {{1, 2}, {1, 3}, {2, 3}, {2, 4}, {3, 4}, {3, 5}, {4, 5}, {4, 6}, {5, 6}};
// 求解顶点覆盖问题
set<int> cover = vertexCover(edges);
// 输出结果
cout << "顶点覆盖集合为:";
for (auto v : cover) {
cout << v << " ";
}
cout << endl;
return 0;
}
```
以上代码中,我们使用了`vector`来存储图的边,使用`set`来存储顶点覆盖集合。算法的核心部分是一个`while`循环,每次从边集合中取出一条边`(u, v)`,将`u`和`v`加入顶点覆盖集合中,并从边集合中删除所有与`u`或`v`相连的边。循环直到边集合为空。
该算法的时间复杂度为$O(|E|\log|E|)$,其中$|E|$是边的数量。
相关推荐












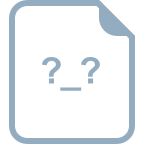
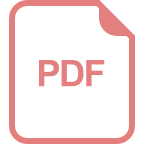