ios用openSSL实现AES-GCM和ECB加密解密
时间: 2024-02-01 11:16:13 浏览: 250
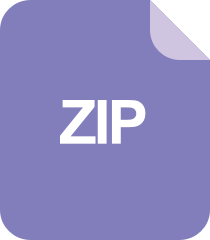
OpenSSL实现AES的ECB和CBC加解密,可一次性加解密任意长度的明文字符串或字节流(QT C++环境)
在iOS中使用OpenSSL实现AES-GCM和ECB加密解密需要以下步骤:
1. 下载OpenSSL库并将其添加到iOS项目中。
2. 导入OpenSSL库头文件:
```objc
#import <openssl/evp.h>
#import <openssl/aes.h>
```
3. 实现AES-GCM加密解密:
```objc
// AES-GCM加密
+ (NSData *)AESGCMEncryptWithKey:(NSData *)key iv:(NSData *)iv aad:(NSData *)aad plainText:(NSData *)plainText tagLength:(NSUInteger)tagLength {
EVP_CIPHER_CTX *ctx;
int len, outlen;
unsigned char *outbuf;
NSData *tag = nil;
NSData *cipherText = nil;
// 初始化EVP_CIPHER_CTX
ctx = EVP_CIPHER_CTX_new();
EVP_EncryptInit_ex(ctx, EVP_aes_256_gcm(), NULL, NULL, NULL);
// 设置key和iv
EVP_CIPHER_CTX_ctrl(ctx, EVP_CTRL_GCM_SET_IVLEN, (int)iv.length, NULL);
EVP_EncryptInit_ex(ctx, NULL, NULL, key.bytes, iv.bytes);
// 设置aad
EVP_EncryptUpdate(ctx, NULL, &len, aad.bytes, (int)aad.length);
// 加密明文
outbuf = malloc(plainText.length + AES_BLOCK_SIZE);
EVP_EncryptUpdate(ctx, outbuf, &len, plainText.bytes, (int)plainText.length);
cipherText = [NSData dataWithBytes:outbuf length:len];
free(outbuf);
// 获取tag
outbuf = malloc(tagLength);
EVP_EncryptFinal_ex(ctx, outbuf, &outlen);
tag = [NSData dataWithBytes:outbuf length:outlen];
free(outbuf);
// 释放EVP_CIPHER_CTX
EVP_CIPHER_CTX_free(ctx);
// 返回加密结果和tag
NSMutableData *resultData = [NSMutableData dataWithData:cipherText];
[resultData appendData:tag];
return resultData;
}
// AES-GCM解密
+ (NSData *)AESGCMDecryptWithKey:(NSData *)key iv:(NSData *)iv aad:(NSData *)aad cipherText:(NSData *)cipherText tagLength:(NSUInteger)tagLength {
EVP_CIPHER_CTX *ctx;
int len, outlen;
unsigned char *outbuf;
NSData *tag = nil;
NSData *plainText = nil;
// 初始化EVP_CIPHER_CTX
ctx = EVP_CIPHER_CTX_new();
EVP_DecryptInit_ex(ctx, EVP_aes_256_gcm(), NULL, NULL, NULL);
// 设置key和iv
EVP_CIPHER_CTX_ctrl(ctx, EVP_CTRL_GCM_SET_IVLEN, (int)iv.length, NULL);
EVP_DecryptInit_ex(ctx, NULL, NULL, key.bytes, iv.bytes);
// 设置aad
EVP_DecryptUpdate(ctx, NULL, &len, aad.bytes, (int)aad.length);
// 解密密文
outbuf = malloc(cipherText.length);
EVP_DecryptUpdate(ctx, outbuf, &len, cipherText.bytes, (int)cipherText.length - tagLength);
plainText = [NSData dataWithBytes:outbuf length:len];
free(outbuf);
// 获取tag
tag = [cipherText subdataWithRange:NSMakeRange(cipherText.length - tagLength, tagLength)];
EVP_CIPHER_CTX_ctrl(ctx, EVP_CTRL_GCM_SET_TAG, (int)tag.length, (void *)tag.bytes);
// 验证tag
if (EVP_DecryptFinal_ex(ctx, NULL, &outlen) <= 0) {
return nil; // tag验证失败
}
// 释放EVP_CIPHER_CTX
EVP_CIPHER_CTX_free(ctx);
// 返回解密结果
return plainText;
}
```
4. 实现ECB加密解密:
```objc
// ECB加密
+ (NSData *)ECBEncryptWithKey:(NSData *)key plainText:(NSData *)plainText {
EVP_CIPHER_CTX *ctx;
int len;
unsigned char *outbuf;
NSData *cipherText = nil;
// 初始化EVP_CIPHER_CTX
ctx = EVP_CIPHER_CTX_new();
EVP_EncryptInit_ex(ctx, EVP_aes_256_ecb(), NULL, key.bytes, NULL);
// 加密明文
outbuf = malloc(plainText.length + AES_BLOCK_SIZE);
EVP_EncryptUpdate(ctx, outbuf, &len, plainText.bytes, (int)plainText.length);
cipherText = [NSData dataWithBytes:outbuf length:len];
free(outbuf);
// 释放EVP_CIPHER_CTX
EVP_CIPHER_CTX_free(ctx);
// 返回加密结果
return cipherText;
}
// ECB解密
+ (NSData *)ECBDecryptWithKey:(NSData *)key cipherText:(NSData *)cipherText {
EVP_CIPHER_CTX *ctx;
int len;
unsigned char *outbuf;
NSData *plainText = nil;
// 初始化EVP_CIPHER_CTX
ctx = EVP_CIPHER_CTX_new();
EVP_DecryptInit_ex(ctx, EVP_aes_256_ecb(), NULL, key.bytes, NULL);
// 解密密文
outbuf = malloc(cipherText.length);
EVP_DecryptUpdate(ctx, outbuf, &len, cipherText.bytes, (int)cipherText.length);
plainText = [NSData dataWithBytes:outbuf length:len];
free(outbuf);
// 释放EVP_CIPHER_CTX
EVP_CIPHER_CTX_free(ctx);
// 返回解密结果
return plainText;
}
```
以上就是使用OpenSSL实现AES-GCM和ECB加密解密的步骤,需要注意的是,在iOS13及以上版本中,Apple已经将OpenSSL库废弃,推荐使用自带的CryptoKit框架实现加密解密。
阅读全文
相关推荐
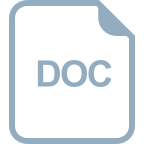
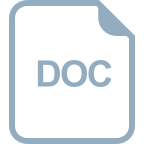

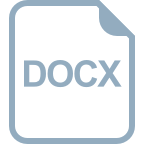
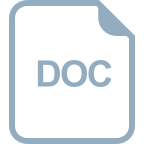
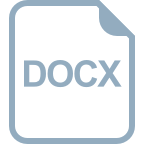


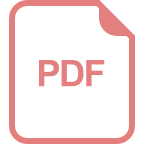
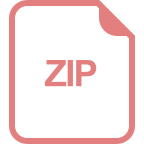
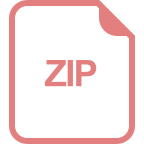
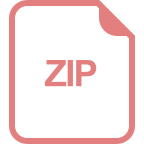
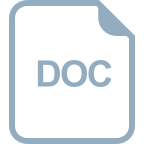




