写一个代码,实现c语言中的线程并发,并有同步机制
时间: 2024-02-12 22:05:31 浏览: 48
好的,以下是一个简单的C语言代码,实现了线程并发,并且使用了互斥锁来实现同步机制:
```c
#include <stdio.h>
#include <stdlib.h>
#include <pthread.h>
#define NUM_THREADS 5
int count = 0;
pthread_mutex_t mutex;
void *print_count(void *thread_id) {
long tid;
tid = (long)thread_id;
for (int i = 0; i < 10; i++) {
pthread_mutex_lock(&mutex);
count++;
printf("Thread #%ld: count = %d\n", tid, count);
pthread_mutex_unlock(&mutex);
}
pthread_exit(NULL);
}
int main() {
pthread_t threads[NUM_THREADS];
int rc;
long t;
pthread_mutex_init(&mutex, NULL);
for (t = 0; t < NUM_THREADS; t++) {
printf("Creating thread %ld\n", t);
rc = pthread_create(&threads[t], NULL, print_count, (void *)t);
if (rc) {
printf("Error: unable to create thread, %d\n", rc);
exit(-1);
}
}
for (t = 0; t < NUM_THREADS; t++) {
pthread_join(threads[t], NULL);
}
pthread_mutex_destroy(&mutex);
printf("Final count: %d\n", count);
pthread_exit(NULL);
}
```
在这个代码中,我们首先定义了一个全局变量 `count`,它会被多个线程共享。然后,我们创建了 `NUM_THREADS` 个线程,并且每个线程会执行 `print_count` 函数。
在 `print_count` 函数中,我们使用了互斥锁 `mutex` 来保证每个线程都可以安全地修改 `count` 变量。具体来说,当一个线程要修改 `count` 变量时,它会先调用 `pthread_mutex_lock` 函数来锁定互斥锁,这样其他线程就无法修改 `count` 变量。当这个线程修改完 `count` 变量后,它会调用 `pthread_mutex_unlock` 函数来释放互斥锁,这样其他线程就可以继续修改 `count` 变量了。
在主函数中,我们使用 `pthread_join` 函数来等待所有线程都执行完毕。最后,我们销毁互斥锁,并且输出最终的 `count` 变量的值。
相关推荐
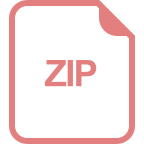
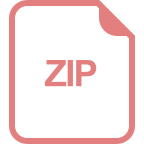
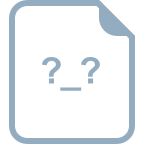














