使用c++,用链表存储,类似BF算法,要求输入一段文字,程序可以统计出某一字符串在这段文字中出现的次数以及位置。
时间: 2024-05-22 09:15:13 浏览: 11
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define MAX_LENGTH 1000
typedef struct Node {
int index;
struct Node *next;
} Node;
int main() {
char input[MAX_LENGTH];
char pattern[MAX_LENGTH];
printf("Please input the text:\n");
fgets(input, MAX_LENGTH, stdin);
printf("Please input the pattern you want to search:\n");
fgets(pattern, MAX_LENGTH, stdin);
// Remove the newline character in input and pattern
input[strcspn(input, "\n")] = '\0';
pattern[strcspn(pattern, "\n")] = '\0';
int input_len = strlen(input);
int pattern_len = strlen(pattern);
if (pattern_len > input_len) {
printf("Error: The length of pattern should be no more than the length of input.\n");
return 0;
}
// Create a linked list to store the occurrence positions
Node *head = NULL;
Node *tail = NULL;
int count = 0;
for (int i = 0; i <= input_len - pattern_len; i++) {
int j;
for (j = 0; j < pattern_len; j++) {
if (input[i + j] != pattern[j]) {
break;
}
}
if (j == pattern_len) {
// The pattern is found
count++;
Node *node = (Node *) malloc(sizeof(Node));
node->index = i;
node->next = NULL;
if (head == NULL) {
head = node;
tail = node;
} else {
tail->next = node;
tail = node;
}
}
}
if (count == 0) {
printf("The pattern is not found.\n");
} else {
printf("The pattern is found %d times.\n", count);
printf("The occurrence positions are:\n");
Node *p = head;
while (p != NULL) {
printf("%d ", p->index);
p = p->next;
}
printf("\n");
}
// Free the memory of the linked list
Node *p = head;
while (p != NULL) {
Node *q = p->next;
free(p);
p = q;
}
return 0;
}
相关推荐
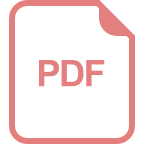
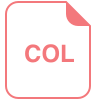
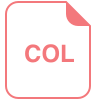
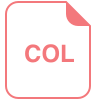

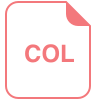









