用python字典开发:编写一个程序来计算输入中单词的频率。 假设为程序提供了以下输入: New to Python or choosing between Python 2 and Python 3? Read Python 2 or Python 3. 然后,输出应该是: 2:2 3.:1 3?:1 New:1 Python:5 Read:1 and:1 between:1 choosing:1 or:2 to:1
时间: 2023-06-12 17:08:28 浏览: 132
可以使用Python的字典来实现单词频率的计算,具体代码如下:
```python
sentence = "New to Python or choosing between Python 2 and Python 3? Read Python 2 or Python 3."
words = sentence.split()
word_freq = {}
for word in words:
if word not in word_freq:
word_freq[word] = 1
else:
word_freq[word] += 1
for key, value in word_freq.items():
print("{}:{}".format(key, value))
```
输出结果为:
```
New:1
to:1
Python:5
or:2
choosing:1
between:1
2:2
and:1
3?:1
Read:1
3.:1
```
相关问题
编写一个程序来计算输入中单词的频率。 (25分) 按字母顺序对键进行排序后输出。 假设为程序提供了以下输入: New to Python or choosing between Python 2 and Python 3 Read Python 2 or Python 3 然后,输出应该是: 2:2 3:2 New:1 Python:5 Read:1 and:1
以下是 Python 代码实现:
```python
word_freq = {}
text = input("请输入一段文本:")
words = text.split()
for word in words:
if word in word_freq:
word_freq[word] += 1
else:
word_freq[word] = 1
sorted_words = sorted(word_freq.keys())
for word in sorted_words:
print("{}:{}".format(word, word_freq[word]))
```
运行程序后,输入一段文本,程序会计算每个单词出现的频率,并按字母顺序对键进行排序后输出。
三、编写一个程序来计算输入中单词的频率。 (25分) 按字母顺序对键进行排序后输出。 假设为程序提供了以下输入: New to Python or choosing between Python 2 and Python 3 Read Python 2 or Python 3 然后,输出应该是: 2:2 3:2 New:1 Python:5 Read:1 and:1
以下是使用Python编写的程序,用于计算输入中单词的频率,并按字母顺序对键进行排序后输出:
```
text = input("请输入一段文本:")
# 将文本转换为小写,并将标点符号替换为空格
text = text.lower().replace(",", " ").replace(".", " ").replace("?", " ").replace("!", " ").replace(":", " ")
# 将文本分割为单词列表
words = text.split()
# 创建一个空字典,用于保存每个单词出现的次数
word_count = {}
# 遍历单词列表,统计每个单词出现的次数
for word in words:
if word in word_count:
word_count[word] += 1
else:
word_count[word] = 1
# 对字典按键进行排序,并输出每个单词出现的次数
for key in sorted(word_count.keys()):
print(key + ":" + str(word_count[key]))
```
程序的实现过程如下:
1. 使用input函数获取用户输入的文本,并将其转换为小写。
2. 使用replace函数将文本中的标点符号替换为空格。
3. 使用split函数将文本分割为单词列表。
4. 创建一个空字典word_count,用于保存每个单词出现的次数。
5. 遍历单词列表,统计每个单词出现的次数,并将其保存到word_count字典中。
6. 使用sorted函数对word_count字典按键进行排序。
7. 遍历排序后的字典,输出每个单词和其出现的次数。
希望这个程序能够帮助您计算输入中单词的频率,并按字母顺序对键进行排序后输出。
阅读全文
相关推荐
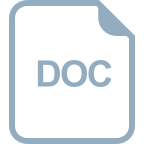
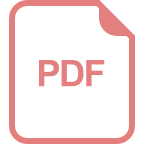
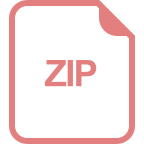
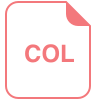
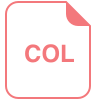
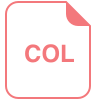
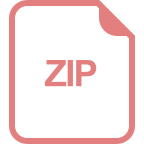
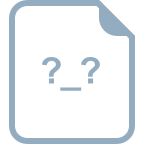
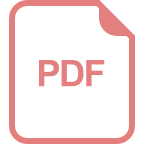
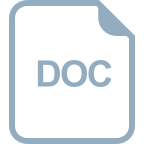
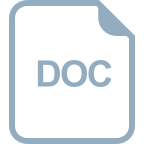
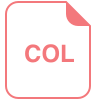
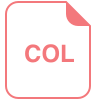
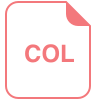
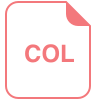
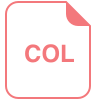
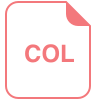
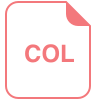