Android studio 音乐列表布局
时间: 2024-05-09 14:20:51 浏览: 9
在Android Studio中,可以使用ListView或RecyclerView来实现音乐列表布局。
ListView布局:
1. 创建一个ListView控件
```
<ListView
android:id="@+id/listView"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:divider="@null"
android:dividerHeight="0dp" />
```
2. 创建一个自定义的列表项布局
```
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:orientation="horizontal"
android:padding="16dp">
<ImageView
android:id="@+id/imageView"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:src="@drawable/music_icon" />
<TextView
android:id="@+id/textViewTitle"
android:layout_width="0dp"
android:layout_weight="1"
android:layout_height="wrap_content"
android:text="Song Title"
android:textSize="18sp"
android:textStyle="bold" />
<TextView
android:id="@+id/textViewArtist"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Artist"
android:textSize="16sp" />
</LinearLayout>
```
3. 在代码中设置适配器和数据源
```
ListView listView = findViewById(R.id.listView);
List<Song> songs = new ArrayList<>();
// 添加歌曲数据
songs.add(new Song("Song 1", "Artist 1", R.drawable.music_icon));
songs.add(new Song("Song 2", "Artist 2", R.drawable.music_icon));
songs.add(new Song("Song 3", "Artist 3", R.drawable.music_icon));
// 创建适配器
SongAdapter adapter = new SongAdapter(this, songs);
// 设置适配器
listView.setAdapter(adapter);
```
4. 创建一个自定义的适配器
```
public class SongAdapter extends ArrayAdapter<Song> {
private Context mContext;
private List<Song> mSongs;
public SongAdapter(Context context, List<Song> songs) {
super(context, 0, songs);
mContext = context;
mSongs = songs;
}
@NonNull
@Override
public View getView(int position, @Nullable View convertView, @NonNull ViewGroup parent) {
View listItem = convertView;
if (listItem == null) {
listItem = LayoutInflater.from(mContext).inflate(R.layout.list_item_song, parent, false);
}
Song song = mSongs.get(position);
ImageView imageView = listItem.findViewById(R.id.imageView);
imageView.setImageResource(song.getImageResource());
TextView textViewTitle = listItem.findViewById(R.id.textViewTitle);
textViewTitle.setText(song.getTitle());
TextView textViewArtist = listItem.findViewById(R.id.textViewArtist);
textViewArtist.setText(song.getArtist());
return listItem;
}
}
```
RecyclerView布局:
1. 创建一个RecyclerView控件
```
<androidx.recyclerview.widget.RecyclerView
android:id="@+id/recyclerView"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:divider="@null"
android:dividerHeight="0dp" />
```
2. 创建一个自定义的列表项布局(与ListView相同)
```
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:orientation="horizontal"
android:padding="16dp">
<ImageView
android:id="@+id/imageView"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:src="@drawable/music_icon" />
<TextView
android:id="@+id/textViewTitle"
android:layout_width="0dp"
android:layout_weight="1"
android:layout_height="wrap_content"
android:text="Song Title"
android:textSize="18sp"
android:textStyle="bold" />
<TextView
android:id="@+id/textViewArtist"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Artist"
android:textSize="16sp" />
</LinearLayout>
```
3. 在代码中设置适配器和数据源(与ListView相同)
```
RecyclerView recyclerView = findViewById(R.id.recyclerView);
List<Song> songs = new ArrayList<>();
// 添加歌曲数据
songs.add(new Song("Song 1", "Artist 1", R.drawable.music_icon));
songs.add(new Song("Song 2", "Artist 2", R.drawable.music_icon));
songs.add(new Song("Song 3", "Artist 3", R.drawable.music_icon));
// 创建适配器
SongAdapter adapter = new SongAdapter(this, songs);
// 设置适配器
recyclerView.setAdapter(adapter);
```
4. 创建一个自定义的适配器(与ListView相同,只需将继承类从ArrayAdapter改为RecyclerView.Adapter)
```
public class SongAdapter extends RecyclerView.Adapter<SongAdapter.ViewHolder> {
private Context mContext;
private List<Song> mSongs;
public SongAdapter(Context context, List<Song> songs) {
mContext = context;
mSongs = songs;
}
@NonNull
@Override
public ViewHolder onCreateViewHolder(@NonNull ViewGroup parent, int viewType) {
View view = LayoutInflater.from(mContext).inflate(R.layout.list_item_song, parent, false);
return new ViewHolder(view);
}
@Override
public void onBindViewHolder(@NonNull ViewHolder holder, int position) {
Song song = mSongs.get(position);
holder.imageView.setImageResource(song.getImageResource());
holder.textViewTitle.setText(song.getTitle());
holder.textViewArtist.setText(song.getArtist());
}
@Override
public int getItemCount() {
return mSongs.size();
}
public class ViewHolder extends RecyclerView.ViewHolder {
ImageView imageView;
TextView textViewTitle;
TextView textViewArtist;
public ViewHolder(@NonNull View itemView) {
super(itemView);
imageView = itemView.findViewById(R.id.imageView);
textViewTitle = itemView.findViewById(R.id.textViewTitle);
textViewArtist = itemView.findViewById(R.id.textViewArtist);
}
}
}
```
相关推荐
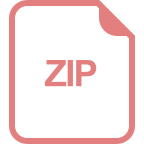
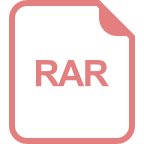














