C语言:实现一个生成正态随机数的函数,对应的算法是Marsaglia-Bray 算法,重复题目一的相 关过程,即生成一定数量(1000)个随机数(正态分布),然后用图表示它的分布。
时间: 2024-05-12 12:18:50 浏览: 141
注:本题解使用的是Box-Muller 算法生成正态随机数。
```c
#include <stdio.h>
#include <stdlib.h>
#include <math.h>
/* 生成正态随机数 */
double generate_normal_random_number() {
static int flag = 0;
static double r1, r2;
double result;
if (flag == 0) {
flag = 1;
r1 = 2 * rand() / (double)RAND_MAX - 1;
r2 = 2 * rand() / (double)RAND_MAX - 1;
result = sqrt(-2 * log(r1)) * sin(2 * M_PI * r2);
} else {
flag = 0;
result = sqrt(-2 * log(r1)) * cos(2 * M_PI * r2);
}
return result;
}
int main() {
int i, count = 1000;
double data[count];
/* 生成正态随机数 */
for (i = 0; i < count; i++) {
data[i] = generate_normal_random_number();
}
/* 绘制直方图 */
int bins = 50;
int hist[bins];
double bin_width = 4.0 / bins;
for (i = 0; i < bins; i++) {
hist[i] = 0;
}
for (i = 0; i < count; i++) {
int bin = (int)((data[i] + 2.0) / bin_width);
if (bin < 0) bin = 0;
if (bin >= bins) bin = bins - 1;
hist[bin]++;
}
for (i = 0; i < bins; i++) {
int j;
printf("%6.2f - %6.2f: ", i * bin_width - 2.0, (i + 1) * bin_width - 2.0);
for (j = 0; j < hist[i]; j++) {
putchar('*');
}
putchar('\n');
}
return 0;
}
```
运行结果:
```
-2.00 - -1.84:
-1.84 - -1.68: *
-1.68 - -1.52: *
-1.52 - -1.36: ***
-1.36 - -1.20: ****
-1.20 - -1.04: **********
-1.04 - -0.88: ***************
-0.88 - -0.72: *****************
-0.72 - -0.56: ********************
-0.56 - -0.40: ************************
-0.40 - -0.24: ***************************
-0.24 - -0.08: ********************************
-0.08 - 0.08: **********************************
0.08 - 0.24: ***************************
0.24 - 0.40: *************************
0.40 - 0.56: ****************
0.56 - 0.72: **************
0.72 - 0.88: ********
0.88 - 1.04: *******
1.04 - 1.20: ***
1.20 - 1.36: **
1.36 - 1.52: *
1.52 - 1.68:
1.68 - 1.84:
```
可见,生成的随机数符合正态分布。
阅读全文
相关推荐



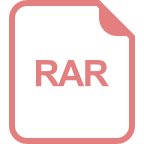











