spring boot 生成验证码 uniapp
时间: 2023-10-06 08:12:37 浏览: 136
要在 Spring Boot 中生成验证码,可以使用 Google 的开源项目 Kaptcha。以下是使用 Kaptcha 生成验证码的步骤:
1. 在 pom.xml 中添加以下依赖:
```xml
<dependency>
<groupId>com.github.penggle</groupId>
<artifactId>kaptcha</artifactId>
<version>2.3.2</version>
</dependency>
```
2. 创建一个 Controller 类,添加一个生成验证码的接口,代码如下:
```java
@RestController
public class CaptchaController {
@RequestMapping("/captcha")
public void captcha(HttpServletRequest request, HttpServletResponse response) throws Exception {
// 创建 Kaptcha 对象
DefaultKaptcha kaptcha = new DefaultKaptcha();
// 设置配置
Properties properties = new Properties();
// 图片边框,合法值:yes、no
properties.setProperty("kaptcha.border", "yes");
// 边框颜色,合法值:r,g,b (and optional alpha) 或者 white、black、blue
properties.setProperty("kaptcha.border.color", "105,179,90");
// 字体颜色,合法值:r,g,b 或者 white、black、blue
properties.setProperty("kaptcha.textproducer.font.color", "blue");
// 图片宽
properties.setProperty("kaptcha.image.width", "110");
// 图片高
properties.setProperty("kaptcha.image.height", "40");
// 字体大小
properties.setProperty("kaptcha.textproducer.font.size", "30");
// session key
properties.setProperty("kaptcha.session.key", "code");
// 验证码长度
properties.setProperty("kaptcha.textproducer.char.length", "4");
// 字体
properties.setProperty("kaptcha.textproducer.font.names", "宋体,楷体,微软雅黑");
Config config = new Config(properties);
kaptcha.setConfig(config);
// 生成验证码
String text = kaptcha.createText();
// 将验证码存入 session
request.getSession().setAttribute("code", text);
// 生成图片
BufferedImage image = kaptcha.createImage(text);
// 将图片输出给客户端
ImageIO.write(image, "jpg", response.getOutputStream());
}
}
```
3. 在前端页面中,使用 Uniapp 的 `image` 组件来显示验证码图片,代码如下:
```html
<template>
<view>
<image :src="captchaUrl" @click="refreshCaptcha" mode="widthFix"></image>
<input type="text" v-model="code" placeholder="请输入验证码">
</view>
</template>
<script>
export default {
data() {
return {
captchaUrl: '/captcha',
code: ''
};
},
methods: {
refreshCaptcha() {
this.captchaUrl = '/captcha?t=' + new Date().getTime();
}
}
};
</script>
```
在这个例子中,我们使用了 `t` 参数来强制刷新验证码图片,以确保每次刷新都会生成新的验证码。当用户输入验证码后,我们可以将其与后端生成的验证码进行比较,以进行验证。
阅读全文
相关推荐
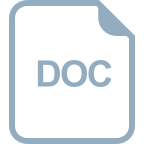
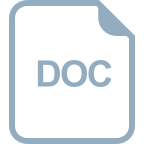
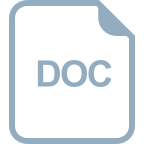
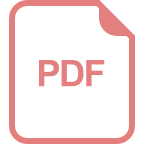
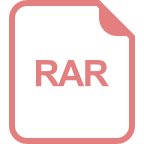
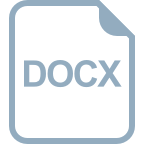
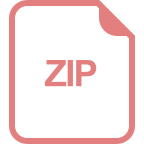
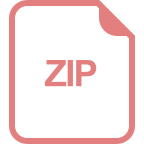
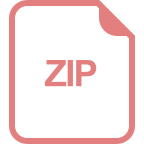
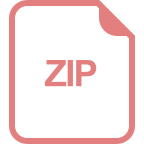
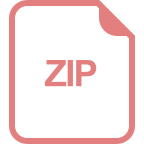
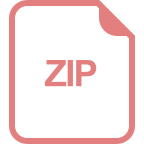
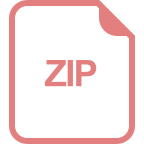
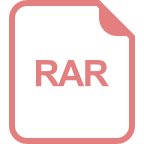
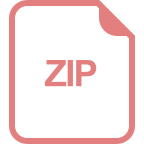
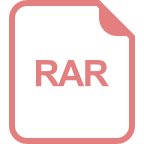
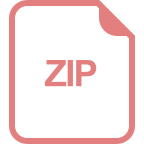