用python写一个考试系统,连接数据库可以实现试题更新,实时打分,智能判卷,并输出分析报告,最后将结果存储到数据库
时间: 2023-11-02 11:04:54 浏览: 82
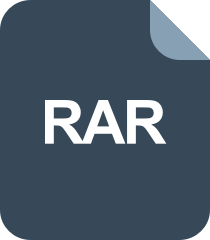
python图像处理- 辅助教学考试答题卡判卷案例
好的,这个题目比较大,我尝试给出一个基本的框架,具体实现需要根据具体需求进行调整。
首先,我们需要一个数据库来存储试题和学生答卷的信息。可以使用 SQLite、MySQL、PostgreSQL 等数据库。这里以 SQLite 为例,使用 Python 内置的 sqlite3 模块进行数据库操作。
创建考试系统的数据库表结构,可以包含以下表:
- 试题表(questions):包含试题编号、题目、选项、答案等信息。
- 学生答卷表(answers):包含学生编号、试题编号、答案、得分等信息。
接下来,我们需要编写程序来实现试题更新、试卷打分、智能判卷和输出分析报告等功能。可以按照以下步骤进行:
1. 连接数据库
使用 sqlite3 模块连接 SQLite 数据库,获取数据库游标对象。
```python
import sqlite3
conn = sqlite3.connect('exam.db')
cursor = conn.cursor()
```
2. 更新试题
从文件或其他来源读取试题信息,然后将试题信息插入到数据库中的试题表中。
```python
def update_questions(file_path):
with open(file_path, 'r') as f:
# 读取试题信息
for line in f:
# 解析试题信息
question, options, answer = parse_question(line)
# 插入试题信息到数据库
cursor.execute("INSERT INTO questions (question, options, answer) VALUES (?, ?, ?)", (question, options, answer))
# 提交数据库事务
conn.commit()
```
3. 生成试卷
根据试题表中的信息,生成一份试卷,并将试卷输出到文件或其他途径。可以根据需要设置试卷的难度、题目数量等参数。
```python
def generate_exam(difficulty, num_questions, file_path):
# 根据难度和题目数量筛选试题
cursor.execute("SELECT * FROM questions WHERE difficulty = ? LIMIT ?", (difficulty, num_questions))
questions = cursor.fetchall()
with open(file_path, 'w') as f:
# 遍历试题,输出到文件
for q in questions:
f.write(q[1] + '\n') # 输出题目
f.write(q[2] + '\n') # 输出选项
f.write('\n') # 输出空行
```
4. 打分和判卷
读取学生答卷,然后根据试题表中的答案进行打分,并将得分和答案插入到数据库的学生答卷表中。
```python
def grade_exam(file_path, student_id):
with open(file_path, 'r') as f:
# 读取学生答卷信息
for i, line in enumerate(f):
if i % 3 == 0: # 题目
question = line.strip()
elif i % 3 == 1: # 选项
options = line.strip()
else: # 答案
answer = line.strip()
# 查询试题答案,进行比较并计算得分
cursor.execute("SELECT answer FROM questions WHERE question = ?", (question,))
correct_answer = cursor.fetchone()[0]
score = 1 if answer == correct_answer else 0
# 插入学生答卷信息到数据库
cursor.execute("INSERT INTO answers (student_id, question, answer, score) VALUES (?, ?, ?, ?)", (student_id, question, answer, score))
# 提交数据库事务
conn.commit()
```
5. 输出分析报告
根据学生答卷表中的信息,输出一份分析报告,包括学生的得分、答对和答错的题目数量等信息。
```python
def generate_report(student_id):
# 查询学生答卷信息
cursor.execute("SELECT * FROM answers WHERE student_id = ?", (student_id,))
answers = cursor.fetchall()
num_correct = 0
num_wrong = 0
total_score = 0
for a in answers:
if a[4] == 1: # 答对
num_correct += 1
total_score += 1
else: # 答错
num_wrong += 1
print(a[2], a[3], a[4]) # 输出题目、学生答案、得分
print('Total score:', total_score)
print('Number correct:', num_correct)
print('Number wrong:', num_wrong)
```
6. 关闭数据库连接
```python
conn.close()
```
这个考试系统的实现还有很多细节需要考虑和完善,比如如何解析试题信息、如何设置试卷难度、如何生成学生编号等等。这里只是给出一个基本的框架,希望能对你有所帮助。
阅读全文
相关推荐
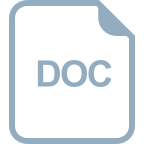
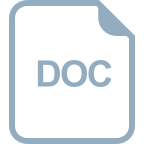
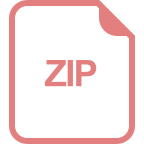
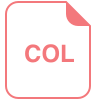
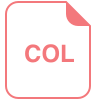
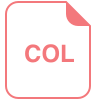
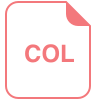
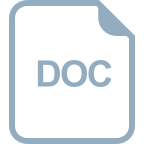
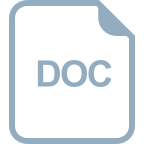
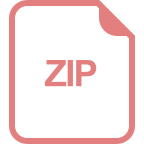
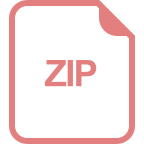
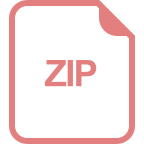
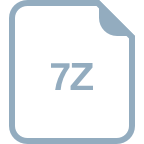
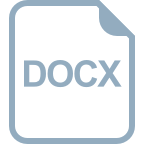