import http.clientfrom html.parser import HTMLParserimport argparsefrom concurrent.futures import ThreadPoolExecutorimport threadingprefix = "save/"readed_path = set()cur_path = []new_path = []lock = threading.Lock()cond=threading.Condition()class MyHttpParser(HTMLParser): def __init__(self): HTMLParser.__init__(self) self.tag = [] self.href = "" self.txt = "" def handle_starttag(self, tag, attrs): self.tag.append(tag) # print("start tag in list :" + str(self.tag)) if tag == "a": for att in attrs: if att[0] == 'href': self.href = att[1] def handle_endtag(self, tag): if tag == "a" and len(self.tag) > 2 and self.tag[-2] == "div": print("in div, link txt is %s ." % self.txt) print("in div, link url is %s ." % self.href) if not self.href in readed_path: readed_path.add(self.href) lock.acquire() new_path.append(self.href) lock.release() # print("end tag in list :" + str(self.tag)) self.tag.pop(-1) def handle_data(self, data): if len(self.tag) >= 1 and self.tag[-1] == "a": self.txt = datadef LoadHtml(path, file_path): if len(file_path) == 0: file_path = "/" conn = http.client.HTTPConnection(path) try: conn.request("GET", file_path) response = conn.getresponse() print(response.status, response.reason, response.version) data = response.read().decode("utf-8") if response.status == 301: data = response.getheader("Location") lock.acquire() new_path.append(data) lock.release() data = "" #print(data) conn.close() return data except Exception as e: print(e.args)怎么设置文件的保存路径
时间: 2024-02-14 18:05:30 浏览: 70
可以在代码中添加一个全局变量 `prefix`,然后在 `LoadHtml()` 函数中拼接上该路径,如下所示:
```python
def LoadHtml(path, file_path):
if len(file_path) == 0:
file_path = "/"
conn = http.client.HTTPConnection(path)
try:
conn.request("GET", file_path)
response = conn.getresponse()
print(response.status, response.reason, response.version)
data = response.read().decode("utf-8")
if response.status == 301:
data = response.getheader("Location")
lock.acquire()
new_path.append(data)
lock.release()
data = ""
# 保存文件
file_name = file_path.replace("/", "_") + ".html"
file_path_with_prefix = prefix + file_name
with open(file_path_with_prefix, "w", encoding="utf-8") as f:
f.write(data)
conn.close()
return data
except Exception as e:
print(e.args)
```
这里将文件名设为 URL 中的路径部分(不含域名)用下划线连接起来,然后添加前缀 `prefix`,最终形成完整的文件路径。在保存文件时,使用 `with open()` 语句打开文件,确保文件关闭。
阅读全文
相关推荐
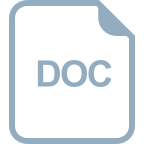
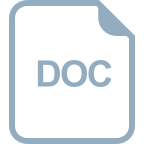
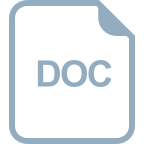



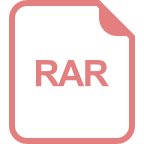
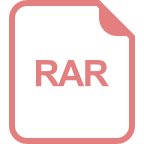
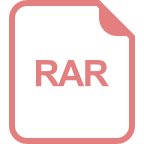
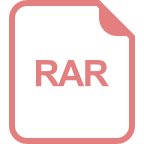
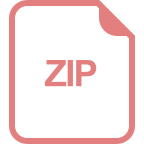
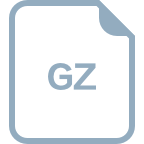
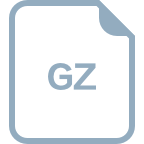
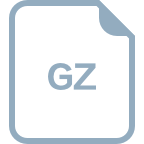
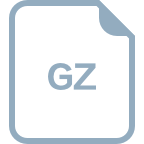
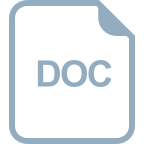
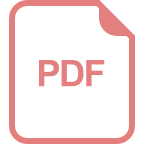
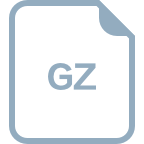