C语言 打开串口
时间: 2023-08-01 14:13:11 浏览: 56
打开串口需要使用C语言中的系统调用函数,具体步骤如下:
1. 引入头文件
```c
#include <stdio.h>
#include <fcntl.h>
#include <termios.h>
#include <unistd.h>
```
2. 定义串口参数
```c
struct termios options;
```
3. 打开串口设备
```c
int fd = open("/dev/ttyUSB0", O_RDWR | O_NOCTTY | O_NDELAY);
```
其中,`/dev/ttyUSB0`是串口设备的文件路径,`O_RDWR`表示以读写方式打开文件,`O_NOCTTY`表示不将该设备作为进程的控制终端,`O_NDELAY`表示非阻塞模式。
4. 配置串口参数
```c
tcgetattr(fd, &options);
cfsetispeed(&options, B9600);
cfsetospeed(&options, B9600);
options.c_cflag |= CLOCAL;
options.c_cflag |= CREAD;
options.c_cflag &= ~CSIZE;
options.c_cflag |= CS8;
options.c_cflag &= ~PARENB;
options.c_cflag &= ~CSTOPB;
options.c_cflag &= ~CRTSCTS;
tcsetattr(fd, TCSANOW, &options);
```
其中,`cfsetispeed`和`cfsetospeed`设置串口的波特率,`CLOCAL`表示忽略调制解调器线路状态,`CREAD`表示使用读取功能,`CS8`表示使用8个数据位,`PARENB`表示不使用奇偶校验,`CSTOPB`表示使用1个停止位,`CRTSCTS`表示不使用硬件流控。
5. 关闭串口设备
```c
close(fd);
```
完整代码如下:
```c
#include <stdio.h>
#include <fcntl.h>
#include <termios.h>
#include <unistd.h>
int main()
{
int fd;
struct termios options;
fd = open("/dev/ttyUSB0", O_RDWR | O_NOCTTY | O_NDELAY);
if (fd == -1)
{
printf("Open serial port failed!\n");
return -1;
}
tcgetattr(fd, &options);
cfsetispeed(&options, B9600);
cfsetospeed(&options, B9600);
options.c_cflag |= CLOCAL;
options.c_cflag |= CREAD;
options.c_cflag &= ~CSIZE;
options.c_cflag |= CS8;
options.c_cflag &= ~PARENB;
options.c_cflag &= ~CSTOPB;
options.c_cflag &= ~CRTSCTS;
tcsetattr(fd, TCSANOW, &options);
close(fd);
return 0;
}
```
相关推荐
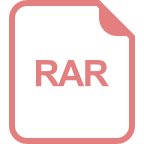














