java写RPC客户端服务端
时间: 2023-10-18 14:09:49 浏览: 152
RPC(Remote Procedure Call)是一种远程过程调用协议。在Java中,可以使用一些框架来实现RPC的客户端和服务端。
常见的Java RPC框架有:
1. Dubbo:阿里巴巴开源的RPC框架,支持服务治理和负载均衡等功能。
2. gRPC:Google开源的RPC框架,使用Protocol Buffers作为序列化协议,支持多语言。
3. Thrift:Facebook开源的跨语言RPC框架,支持多种数据格式和传输协议。
下面以Dubbo为例,介绍如何实现Java RPC客户端和服务端:
1. 定义服务接口
```
public interface HelloService {
String sayHello(String name);
}
```
2. 实现服务接口
```
public class HelloServiceImpl implements HelloService {
public String sayHello(String name) {
return "Hello, " + name;
}
}
```
3. 配置Dubbo服务
```
<dubbo:service interface="com.example.HelloService" ref="helloService" />
<bean id="helloService" class="com.example.HelloServiceImpl" />
```
4. 编写客户端代码
```
public class HelloClient {
public static void main(String[] args) {
ApplicationConfig application = new ApplicationConfig();
application.setName("hello-client");
ReferenceConfig<HelloService> reference = new ReferenceConfig<>();
reference.setApplication(application);
reference.setInterface(HelloService.class);
reference.setUrl("dubbo://localhost:20880/com.example.HelloService");
HelloService helloService = reference.get();
String result = helloService.sayHello("world");
System.out.println(result);
}
}
```
5. 编写服务端代码
```
public class HelloServer {
public static void main(String[] args) throws Exception {
ApplicationConfig application = new ApplicationConfig();
application.setName("hello-server");
RegistryConfig registry = new RegistryConfig();
registry.setAddress("zookeeper://localhost:2181");
ServiceConfig<HelloService> service = new ServiceConfig<>();
service.setApplication(application);
service.setRegistry(registry);
service.setInterface(HelloService.class);
service.setRef(new HelloServiceImpl());
service.export();
System.in.read();
}
}
```
以上是使用Dubbo实现Java RPC客户端和服务端的基本步骤,具体实现细节可以参考官方文档或相关教程。
阅读全文
相关推荐















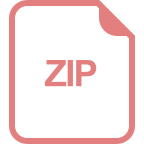