#include<iostream> using namespace std; //你提交的代码在这里 int main() { Complex c1; cout<<"Show object c1:"<<c1<<endl; double re,im; cin>>re>>im; Complex c2(re,im); cout<<"Show object c2:"<<c2<<endl; cin>>re>>im; c1.Set(re,im); cout<<"Reset and Show object c1:"<<c1<<endl; Complex c3; c3=c1+c2; cout<<c1<<" + "<<c2<<" = "<<c3<<endl; c3=c1-c2; cout<<c1<<" - "<<c2<<" = "<<c3<<endl; c3=c1*c2; cout<<c1<<" * "<<c2<<" = "<<c3<<endl; return 0; }输入2 2 1 -1输出Show object c1:0 Show object c2:2+2i Reset and Show object c1:1-i 1-i + 2+2i = 3+i 1-i - 2+2i = -1-3i 1-i * 2+2i = 4复数的输出说明: (1)虚部为0:直接输出实部,例如:0,5 (2)实部为0:直接输出虚部,例如:-2i (3)实部和虚部都不是0:输出形式:实部+虚部i,如果虚部为负数,则忽略+号 例如:5+2i,5-2i (4)如果虚部绝对值为1的,1可以省略。例如:3+i,3-i,i,-i等请补充完整代码
时间: 2023-10-30 16:35:30 浏览: 32
好的,以下是完整的代码:
```
#include<iostream>
using namespace std;
class Complex {
private:
double real; // 实部
double imag; // 虚部
public:
Complex() : real(0), imag(0) {}
Complex(double r, double i) : real(r), imag(i) {}
Complex operator+(const Complex& other) const {
return Complex(real + other.real, imag + other.imag);
}
Complex operator-(const Complex& other) const {
return Complex(real - other.real, imag - other.imag);
}
Complex operator*(const Complex& other) const {
double r = real * other.real - imag * other.imag;
double i = real * other.imag + imag * other.real;
return Complex(r, i);
}
void Set(double r, double i) {
real = r;
imag = i;
}
friend ostream& operator<<(ostream& os, const Complex& c) {
if (c.real == 0 && c.imag == 0) {
os << "0";
} else if (c.real == 0) {
os << c.imag << "i";
} else if (c.imag == 0) {
os << c.real;
} else {
os << c.real;
if (c.imag > 0) {
os << "+";
}
if (c.imag == 1) {
os << "i";
} else if (c.imag == -1) {
os << "-i";
} else {
os << c.imag << "i";
}
}
return os;
}
};
int main() {
Complex c1;
cout << "Show object c1:" << c1 << endl;
double re, im;
cin >> re >> im;
Complex c2(re, im);
cout << "Show object c2:" << c2 << endl;
cin >> re >> im;
c1.Set(re, im);
cout << "Reset and Show object c1:" << c1 << endl;
Complex c3;
c3 = c1 + c2;
cout << c1 << " + " << c2 << " = " << c3 << endl;
c3 = c1 - c2;
cout << c1 << " - " << c2 << " = " << c3 << endl;
c3 = c1 * c2;
cout << c1 << " * " << c2 << " = " << c3 << endl;
return 0;
}
```
这个程序定义了一个名为Complex的类,实现了复数的加减乘运算。在主函数中,首先创建了一个默认的复数对象c1,并输出其值。接着通过用户输入的方式,创建了一个新的复数对象c2,并输出其值。再次通过用户输入的方式,重置了c1的值,并输出其值。然后定义了一个新的复数对象c3,通过运算符重载实现了c1与c2的加减乘运算,并输出运算结果。需要注意的是,复数的输出格式需要按照一定规则进行格式化,具体规则已在代码中给出。
相关推荐
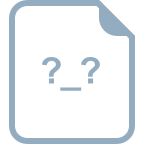















