用c++编辑一个三百行的学生选课系统
时间: 2023-11-06 20:04:12 浏览: 94
好的,下面是一个简单的学生选课系统的代码示例,仅供参考:
```c++
#include <iostream>
#include <fstream>
#include <vector>
#include <string>
using namespace std;
// 定义学生和课程的结构体
struct Student {
string name;
string id;
vector<string> courses;
};
struct Course {
string name;
string id;
int capacity;
int selected;
};
// 学生信息和课程信息的存储文件
const string STUDENT_FILE = "students.txt";
const string COURSE_FILE = "courses.txt";
// 读取学生和课程的信息
void readStudents(vector<Student>& students) {
ifstream fin(STUDENT_FILE);
if (!fin) {
cout << "Error opening file " << STUDENT_FILE << endl;
return;
}
string name, id;
while (fin >> name >> id) {
Student s;
s.name = name;
s.id = id;
string course;
while (fin >> course) {
s.courses.push_back(course);
}
students.push_back(s);
}
fin.close();
}
void readCourses(vector<Course>& courses) {
ifstream fin(COURSE_FILE);
if (!fin) {
cout << "Error opening file " << COURSE_FILE << endl;
return;
}
string name, id;
int capacity, selected;
while (fin >> name >> id >> capacity >> selected) {
Course c;
c.name = name;
c.id = id;
c.capacity = capacity;
c.selected = selected;
courses.push_back(c);
}
fin.close();
}
// 保存学生和课程的信息
void saveStudents(const vector<Student>& students) {
ofstream fout(STUDENT_FILE);
if (!fout) {
cout << "Error opening file " << STUDENT_FILE << endl;
return;
}
for (const auto& s : students) {
fout << s.name << " " << s.id << " ";
for (const auto& c : s.courses) {
fout << c << " ";
}
fout << endl;
}
fout.close();
}
void saveCourses(const vector<Course>& courses) {
ofstream fout(COURSE_FILE);
if (!fout) {
cout << "Error opening file " << COURSE_FILE << endl;
return;
}
for (const auto& c : courses) {
fout << c.name << " " << c.id << " " << c.capacity << " " << c.selected << endl;
}
fout.close();
}
// 显示学生和课程的信息
void showStudents(const vector<Student>& students) {
cout << "Name\tID\tCourses" << endl;
for (const auto& s : students) {
cout << s.name << "\t" << s.id << "\t";
for (const auto& c : s.courses) {
cout << c << " ";
}
cout << endl;
}
}
void showCourses(const vector<Course>& courses) {
cout << "Name\tID\tCapacity\tSelected" << endl;
for (const auto& c : courses) {
cout << c.name << "\t" << c.id << "\t" << c.capacity << "\t\t" << c.selected << endl;
}
}
// 学生选课和退课的操作
void selectCourse(vector<Student>& students, vector<Course>& courses, const string& studentId, const string& courseId) {
// 查找学生和课程
auto s = find_if(students.begin(), students.end(), [studentId](const Student& s) { return s.id == studentId; });
auto c = find_if(courses.begin(), courses.end(), [courseId](const Course& c) { return c.id == courseId; });
if (s == students.end() || c == courses.end()) {
cout << "Invalid student ID or course ID" << endl;
return;
}
// 检查课程容量和学生是否已选该课程
if (c->selected >= c->capacity) {
cout << "Course is full" << endl;
return;
}
if (find(s->courses.begin(), s->courses.end(), courseId) != s->courses.end()) {
cout << "Student has selected this course" << endl;
return;
}
// 更新学生和课程的信息
s->courses.push_back(courseId);
c->selected++;
saveStudents(students);
saveCourses(courses);
cout << "Select course successfully" << endl;
}
void dropCourse(vector<Student>& students, vector<Course>& courses, const string& studentId, const string& courseId) {
// 查找学生和课程
auto s = find_if(students.begin(), students.end(), [studentId](const Student& s) { return s.id == studentId; });
auto c = find_if(courses.begin(), courses.end(), [courseId](const Course& c) { return c.id == courseId; });
if (s == students.end() || c == courses.end()) {
cout << "Invalid student ID or course ID" << endl;
return;
}
// 检查学生是否选了该课程
auto it = find(s->courses.begin(), s->courses.end(), courseId);
if (it == s->courses.end()) {
cout << "Student has not selected this course" << endl;
return;
}
// 更新学生和课程的信息
s->courses.erase(it);
c->selected--;
saveStudents(students);
saveCourses(courses);
cout << "Drop course successfully" << endl;
}
// 主函数
int main() {
vector<Student> students;
vector<Course> courses;
readStudents(students);
readCourses(courses);
int choice;
string studentId, courseId;
while (true) {
cout << "======== Student Course Selection System ========" << endl;
cout << "1. Show students" << endl;
cout << "2. Show courses" << endl;
cout << "3. Select course" << endl;
cout << "4. Drop course" << endl;
cout << "5. Exit" << endl;
cout << "Enter your choice: ";
cin >> choice;
switch (choice) {
case 1:
showStudents(students);
break;
case 2:
showCourses(courses);
break;
case 3:
cout << "Enter student ID: ";
cin >> studentId;
cout << "Enter course ID: ";
cin >> courseId;
selectCourse(students, courses, studentId, courseId);
break;
case 4:
cout << "Enter student ID: ";
cin >> studentId;
cout << "Enter course ID: ";
cin >> courseId;
dropCourse(students, courses, studentId, courseId);
break;
case 5:
return 0;
default:
cout << "Invalid choice" << endl;
break;
}
}
return 0;
}
```
这份代码实现了学生选课系统的基本功能,包括学生信息和课程信息的录入和查询,学生选课和退课的操作等。需要注意的是,这份代码并不是完整的实现,可能还需要根据具体需求进行修改和完善。
阅读全文
相关推荐




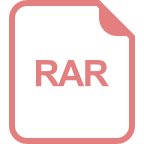












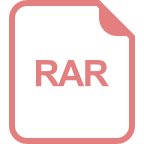
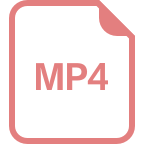