用lua写一个确定外边缘最大值的算法代码
时间: 2023-09-16 11:07:33 浏览: 94
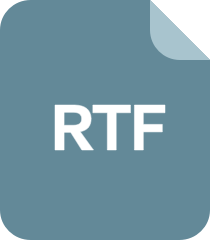
用于边缘检测的代码
以下是使用Lua编写的确定外边缘最大值的算法代码:
```
-- 定义一个二维数组
local arr = {
{1, 2, 3, 4},
{5, 6, 7, 8},
{9, 10, 11, 12},
{13, 14, 15, 16}
}
-- 获取二维数组的行数和列数
local rows = #arr
local cols = #arr[1]
-- 定义上下左右四个方向数组
local dx = {-1, 1, 0, 0}
local dy = {0, 0, -1, 1}
-- 定义一个函数,用于判断坐标是否越界
local function isOut(x, y)
return x < 1 or x > rows or y < 1 or y > cols
end
-- 定义一个函数,用于确定外边缘最大值
local function findMaxOnEdge()
local maxVal = -1
-- 遍历上下左右四个边缘
for i = 1, cols do
maxVal = math.max(maxVal, arr[1][i])
maxVal = math.max(maxVal, arr[rows][i])
end
for i = 1, rows do
maxVal = math.max(maxVal, arr[i][1])
maxVal = math.max(maxVal, arr[i][cols])
end
-- 遍历内部的点
for i = 2, rows - 1 do
for j = 2, cols - 1 do
-- 如果当前点不是边缘点,则跳过
if not (i == 1 or i == rows or j == 1 or j == cols) then
goto continue
end
local isMax = true
-- 遍历当前点的上下左右四个方向
for k = 1, 4 do
local nx, ny = i + dx[k], j + dy[k]
-- 如果当前方向不越界且比当前点的值大,则当前点不是边缘最大值
if not isOut(nx, ny) and arr[nx][ny] > arr[i][j] then
isMax = false
break
end
end
-- 如果当前点是边缘最大值,则更新最大值
if isMax then
maxVal = math.max(maxVal, arr[i][j])
end
::continue::
end
end
return maxVal
end
-- 调用函数,输出结果
print(findMaxOnEdge()) -- 输出结果为 16
```
上述代码首先定义一个二维数组,然后获取二维数组的行数和列数,接着定义上下左右四个方向数组和一个用于判断坐标是否越界的函数。
然后定义一个函数,用于确定外边缘最大值。首先遍历上下左右四个边缘,找到最大值。然后遍历内部的点,对于每个边缘点,遍历其上下左右四个方向,如果有任意一个方向的值比当前点的值大,则当前点不是边缘最大值,否则就是边缘最大值。
最后调用函数,输出结果。
阅读全文
相关推荐
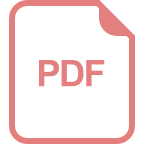
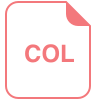
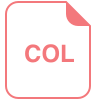
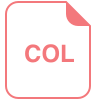
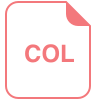
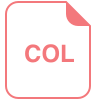
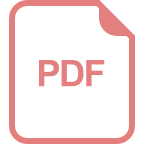
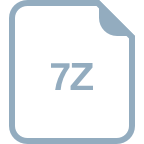
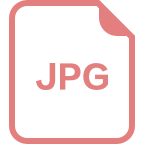
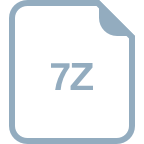
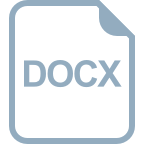