#include <stdio.h> #include <stdlib.h> typedef struct { int key; int value; } Record; void create_table(Record *table, int n) { for (int i = 0; i < n; i++) { printf("请输入第%d个关键字值:", i + 1); scanf("%d", &table[i].key); printf("请输入第%d个关键字值对应的记录值:", i + 1); scanf("%d", &table[i].value); } } void binary_search(Record *table, int n, int target) { int left = 0; int right = n - 1; while (left <= right) { int mid = left + (right - left) / 2; if (table[mid].key == target) { printf("找到记录,键值为:%d,记录值为:%d ", table[mid].key, table[mid].value); return; } else if (table[mid].key < target) { left = mid + 1; } else { right = mid - 1; } } printf("未找到记录,键值为:%d ", target); } int main() { int n; int choice; Record table[100]; while (1) { printf("菜单: "); printf("1. 建立顺序查找表并进行顺序查找 "); printf("2. 建立有序顺序查找表并进行二分查找 "); printf("3. 退出程序 "); printf("请输入您的选择:"); scanf("%d", &choice); switch (choice) { case 1: create_table(table, n); break; case 2: binary_search(table, n, getchar()); // 注意这里需要先用getchar()获取用户输入的换行符,否则会报错 break; case 3: exit(0); default: printf("无效的选择,请重新输入。 "); } } return 0; }
时间: 2024-02-14 13:20:06 浏览: 92
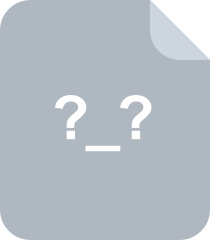
#include <stdio.h>
这段代码是一个简单的顺序查找和二分查找的实现。其中,create_table函数用于创建一个记录表,binary_search函数用于在有序记录表中进行二分查找。在main函数中,通过菜单的方式选择进行哪种操作。
需要注意的是,在调用binary_search函数时,需要先用getchar()获取用户输入的换行符,否则会导致输入错误。
阅读全文
相关推荐
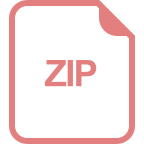

















