C语言 使用zlib解压文件夹
时间: 2023-10-10 08:09:32 浏览: 63
在 C 语言中,可以使用 zlib 库提供的函数对压缩文件进行解压缩。以下是一个使用 zlib 库解压缩文件夹的示例代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <zlib.h>
#define CHUNKSIZE 16384
int main(int argc, char *argv[]) {
if (argc != 2) {
fprintf(stderr, "Usage: %s <compressed_folder.zip>\n", argv[0]);
return 1;
}
char *input_file = argv[1];
gzFile input = gzopen(input_file, "rb");
if (!input) {
fprintf(stderr, "Failed to open input file: %s\n", input_file);
return 1;
}
char *output_folder = "output_folder";
char *command = malloc(strlen("mkdir -p ") + strlen(output_folder) + 1);
sprintf(command, "mkdir -p %s", output_folder);
system(command);
free(command);
char *buffer = malloc(CHUNKSIZE);
int ret;
do {
ret = gzread(input, buffer, CHUNKSIZE);
if (ret < 0) {
fprintf(stderr, "Failed to read from input file: %s\n", input_file);
return 1;
}
char *output_file = malloc(strlen(output_folder) + 1 + CHUNKSIZE);
sprintf(output_file, "%s/%.*s", output_folder, ret - 4, buffer);
FILE *output = fopen(output_file, "wb");
if (!output) {
fprintf(stderr, "Failed to open output file: %s\n", output_file);
free(output_file);
return 1;
}
fwrite(buffer + ret - 4, 1, 4, output);
gzrewind(input);
gzoffset(input);
do {
ret = gzread(input, buffer, CHUNKSIZE);
if (ret < 0) {
fprintf(stderr, "Failed to read from input file: %s\n", input_file);
fclose(output);
remove(output_file);
free(output_file);
return 1;
}
fwrite(buffer, 1, ret, output);
} while (ret == CHUNKSIZE);
fclose(output);
free(output_file);
} while (ret == CHUNKSIZE);
gzclose(input);
free(buffer);
return 0;
}
```
这个示例代码会将压缩文件夹解压缩到当前目录下的 `output_folder` 文件夹中。在解压缩过程中,它会逐个文件地解压缩,并将解压缩后的文件保存到指定的目录中。
需要注意的是,这个示例代码仅支持解压缩 .zip 格式的压缩文件,如果要解压缩其他格式的压缩文件,需要使用其他工具或者库。
相关推荐














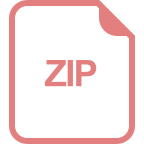