system.out.println(b+a);
时间: 2024-05-27 16:14:55 浏览: 7
This code will result in a compilation error as there is no operator between "b" and "a". It should be written as:
System.out.println("b" + "a");
This will print the string "ba" to the console.
相关问题
解读一下一下java代码int x=7,y=8,z=9; char ch='A'; x++; z--; System.out.println(x==y); System.out.println(y=z--); System.out.println(x==z); System.out.println(ch++=='A'); System.out.println(ch++=='B');
首先,让我们一行一行地解析这段 Java 代码:
```java
int x = 7, y = 8, z = 9;
```
这行代码声明了三个整数变量 x、y 和 z,并分别初始化为 7、8 和 9。
```java
char ch = 'A';
```
这行代码声明了一个字符变量 ch,并初始化为字符 'A'。
```java
x++;
z--;
```
这两行代码分别对变量 x 和 z 进行了自增和自减操作。x++ 表示先使用变量 x 的值,然后再将 x 的值加 1,而 z-- 表示先使用变量 z 的值,然后再将 z 的值减 1。
```java
System.out.println(x == y);
```
这行代码输出了一个布尔值,判断变量 x 是否等于变量 y。在这种情况下,x 和 y 的值分别为 8 和 8,因此输出结果为 true。
```java
System.out.println(y = z--);
```
这行代码输出了一个整数值,并将变量 y 的值更新为变量 z 的值。由于后缀自减运算符 z-- 表示先使用变量 z 的值,然后再将 z 的值减 1,因此输出结果为 9,并将 y 的值更新为 9。
```java
System.out.println(x == z);
```
这行代码输出了一个布尔值,判断变量 x 是否等于变量 z。在这种情况下,x 和 z 的值分别为 8 和 8,因此输出结果为 true。
```java
System.out.println(ch++ == 'A');
```
这行代码输出了一个布尔值,判断变量 ch 自增后的值是否等于字符 'A'。由于后缀自增运算符 ch++ 表示先使用变量 ch 的值,然后再将 ch 的值加 1,因此输出结果为 true。此时变量 ch 的值为 'B',但在判断中使用的是自增前的值 'A'。
```java
System.out.println(ch++ == 'B');
```
这行代码输出了一个布尔值,判断变量 ch 自增后的值是否等于字符 'B'。由于后缀自增运算符 ch++ 表示先使用变量 ch 的值,然后再将 ch 的值加 1,因此输出结果为 false。此时变量 ch 的值为 'C',而不是判断中的字符 'B'。
希望能够帮助你理解这段 Java 代码!如果你还有其他问题,请随时提问。
就是 当 BiCosumer调用了 accept方法便会 使用 这个函数-> System.out.println(a + b);
是的,当你调用BiConsumer的accept方法并传递参数时,它会执行你在Lambda表达式中定义的操作。
回到我之前的例子:
```
BiConsumer<Integer, Integer> sumPrinter = (a, b) -> System.out.println(a + b);
sumPrinter.accept(2, 3); // 输出 5
```
在这个例子中,我们定义了一个BiConsumer类型的变量sumPrinter,它接受两个整数并打印它们的和。然后我们使用这个变量来调用accept方法,并传递两个整数2和3作为参数,这样它就会输出5。
当我们调用sumPrinter.accept(2, 3)时,它会执行Lambda表达式中的操作,即打印两个整数的和。所以最终输出的结果是5。
相关推荐
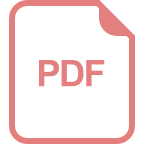
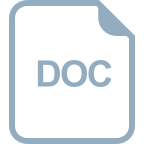
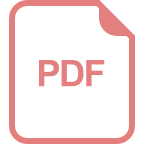












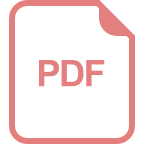