用python写一个办公自动化的软件
时间: 2023-06-13 11:03:15 浏览: 100
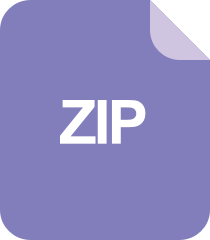
49个Python案例源码(办公自动化-高效办公就靠它了)
好的,下面是一个简单的办公自动化软件的示例,可以用Python语言实现:
```python
import os
import subprocess
import time
def open_file(file_path):
if os.path.exists(file_path):
subprocess.call(['open', file_path])
else:
print("File not found!")
def send_email(to, subject, body):
subprocess.call(['open', 'mailto:%s?subject=%s&body=%s' % (to, subject, body)])
def schedule_meeting(date, time, duration, attendees):
print("Scheduling meeting on %s at %s for %d minutes with attendees: %s" % (date, time, duration, attendees))
def take_notes():
notes = ""
print("Taking notes. Press 'q' to exit.")
while True:
line = input()
if line == 'q':
break
notes += line + "\n"
print(notes)
def main():
while True:
print("What would you like to do?")
print("1. Open a file")
print("2. Send an email")
print("3. Schedule a meeting")
print("4. Take notes")
print("5. Quit")
choice = input("Enter your choice: ")
if choice == '1':
file_path = input("Enter the file path: ")
open_file(file_path)
elif choice == '2':
to = input("To: ")
subject = input("Subject: ")
body = input("Body: ")
send_email(to, subject, body)
elif choice == '3':
date = input("Date (MM/DD/YYYY): ")
time = input("Time (HH:MM AM/PM): ")
duration = int(input("Duration (in minutes): "))
attendees = input("Attendees (comma separated): ")
schedule_meeting(date, time, duration, attendees)
elif choice == '4':
take_notes()
elif choice == '5':
break
else:
print("Invalid choice!")
time.sleep(2)
if __name__ == '__main__':
main()
```
这个办公自动化软件可以执行以下操作:
1. 打开文件
2. 发送电子邮件
3. 安排会议
4. 记笔记
你可以根据自己的需要进行修改和扩展。
阅读全文
相关推荐
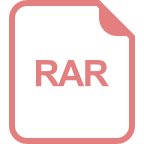
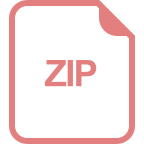















