编写一段c语言代码,不使用Pi库,通过计算按键按下次数来实现控制led流水灯数量
时间: 2024-05-05 11:17:21 浏览: 97
假设按键连接到GPIOA的第0位,LED连接到GPIOB的前4位,以下是一段示例代码:
```c
#include "stm32f10x.h"
int main(void)
{
// 初始化GPIOA和GPIOB
RCC_APB2PeriphClockCmd(RCC_APB2Periph_GPIOA | RCC_APB2Periph_GPIOB, ENABLE);
GPIO_InitTypeDef GPIO_InitStructure;
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_IPU; // 按键连接到GPIOA的第0位,需要设置为输入上拉模式
GPIO_InitStructure.GPIO_Pin = GPIO_Pin_0;
GPIO_Init(GPIOA, &GPIO_InitStructure);
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_Out_PP; // LED连接到GPIOB的前4位,需要设置为推挽输出模式
GPIO_InitStructure.GPIO_Pin = GPIO_Pin_0 | GPIO_Pin_1 | GPIO_Pin_2 | GPIO_Pin_3;
GPIO_Init(GPIOB, &GPIO_InitStructure);
// 初始化按键按下次数为0
uint8_t key_press_count = 0;
while (1)
{
// 判断按键是否按下
if (!GPIO_ReadInputDataBit(GPIOA, GPIO_Pin_0))
{
// 延时一段时间,等待按键抖动结束
for (volatile int i = 0; i < 100000; i++);
// 再次判断按键是否按下,避免误触
if (!GPIO_ReadInputDataBit(GPIOA, GPIO_Pin_0))
{
// 按键按下次数加1
key_press_count++;
// 如果按键按下次数超过4,则重置为0
if (key_press_count > 4)
{
key_press_count = 0;
}
}
}
// 根据按键按下次数控制LED流水灯数量
switch (key_press_count)
{
case 0:
GPIO_ResetBits(GPIOB, GPIO_Pin_0 | GPIO_Pin_1 | GPIO_Pin_2 | GPIO_Pin_3);
break;
case 1:
GPIO_SetBits(GPIOB, GPIO_Pin_0);
GPIO_ResetBits(GPIOB, GPIO_Pin_1 | GPIO_Pin_2 | GPIO_Pin_3);
break;
case 2:
GPIO_SetBits(GPIOB, GPIO_Pin_0 | GPIO_Pin_1);
GPIO_ResetBits(GPIOB, GPIO_Pin_2 | GPIO_Pin_3);
break;
case 3:
GPIO_SetBits(GPIOB, GPIO_Pin_0 | GPIO_Pin_1 | GPIO_Pin_2);
GPIO_ResetBits(GPIOB, GPIO_Pin_3);
break;
case 4:
GPIO_SetBits(GPIOB, GPIO_Pin_0 | GPIO_Pin_1 | GPIO_Pin_2 | GPIO_Pin_3);
break;
}
}
}
```
在上述代码中,我们使用一个变量 `key_press_count` 来记录按键按下的次数,根据不同的次数来控制LED流水灯的数量。当按键按下时,我们需要延时一段时间等待按键抖动结束,然后再次判断按键是否按下,避免误触。当按键按下次数超过4时,我们将其重置为0。
阅读全文
相关推荐
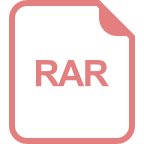
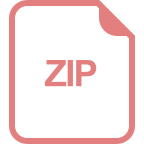
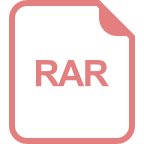
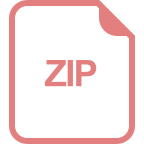
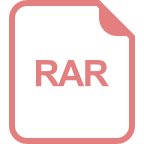
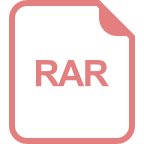
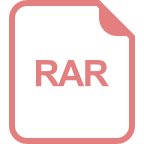
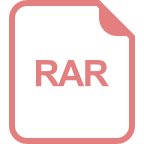
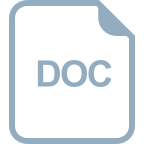
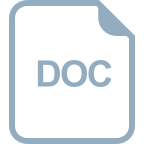
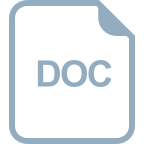