Eclipse小游戏-俄罗斯方块
时间: 2023-10-10 18:09:55 浏览: 74
好的,我可以为您提供一些关于Eclipse小游戏-俄罗斯方块的指导。
首先,您需要安装Eclipse开发环境以及Java开发工具包。然后,您需要根据游戏规则和界面设计开发游戏。
以下是一些可能有用的指导:
1. 游戏规则
俄罗斯方块游戏的规则比较简单,玩家需要控制不同形状的方块下落并拼凑成完整的一行,每拼接一行则消除该行并获得相应的分数。随着游戏难度的增加,方块下落速度也会加快,玩家需要尽快拼接方块以避免游戏结束。
2. 界面设计
游戏的界面设计需要考虑到游戏元素的布局和美观度。通常,游戏界面应该包括游戏区域,得分区域和下一块区域。游戏区域应该包含方块下落的场景,得分区域应该显示当前玩家的得分,下一块区域应该显示下一个方块的形状。
3. 开发过程
开发过程中,您需要使用Java编写游戏逻辑和界面。您可以使用Java Swing或JavaFX等图形用户界面库来实现游戏界面。同时,您需要编写游戏逻辑,包括方块的下落、旋转、移动等操作,以及得分和游戏结束等判定。
希望这些指导对您有所帮助!
相关问题
用eclipse写一个俄罗斯方块
要使用Eclipse编写一个俄罗斯方块游戏,可以按照以下步骤进行:
第一步:创建一个新的Java项目。
在Eclipse中,选择File(文件) -> New(新建) -> Java Project(Java项目),然后输入项目名称并点击Finish(完成)。
第二步:创建游戏窗口。
在项目中创建一个新的Java类,命名为GameWindow(游戏窗口)。在该类中,使用Swing或JavaFX等库来创建一个窗口,并设置其大小和标题。可以选择适当的布局管理器来组织游戏窗口中的元素。
第三步:实现方块类和方块移动。
在项目中创建一个新的Java类,命名为Block(方块)。在该类中,定义方块的属性,如颜色、形状和位置。实现方块移动的方法,如向左、向右和向下移动,以及旋转方块的方法。
第四步:实现游戏逻辑。
在项目中创建一个新的Java类,命名为Game(游戏)。在该类中,定义游戏的属性,如分数和游戏区域。实现游戏开始、暂停和结束的方法,以及处理方块碰撞和消除行的方法。
第五步:实现用户输入和控制。
在GameWindow类中,添加键盘监听器或鼠标监听器来处理用户输入,如移动方块或旋转方块。
第六步:在游戏窗口中显示游戏状态。
在GameWindow类中,使用绘图方法来显示游戏区域和方块。可以在窗口中绘制游戏状态,并在每次游戏状态改变时刷新窗口。
最后一步:测试和调试。
使用Eclipse的调试功能来测试和调试游戏。检查游戏的各个方面是否按预期运行,并修复可能出现的错误和问题。
通过按照以上步骤,您可以使用Eclipse编写一个简单的俄罗斯方块游戏。随着更深入的学习和开发,您还可以添加更多功能和特性,使游戏更加完善和有趣。
eclipse俄罗斯方块
很抱歉,根据提供的引用内容,我无法为您提供关于eclipse实现俄罗斯方块的具体介绍或演示。引用中提到了使用eclipse作为IDE实现的Android俄罗斯方块,但没有提供具体的代码或实现细节。如果您对eclipse实现俄罗斯方块感兴趣,我建议您在搜索引擎中查找相关的教程或示例代码,以便更详细地了解如何使用eclipse实现俄罗斯方块游戏。
相关推荐
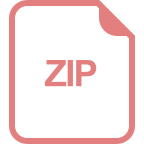
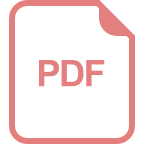












