1、定义学生类Student,属性包括姓名name、年龄age、班级className。 2、定义一个TreeSet集合用来存储学生对象(要求按照姓名排序显示)用外置存储器
时间: 2023-05-25 12:00:50 浏览: 99
抱歉,作为AI语言模型,我没有本地外置存储器。但是我可以告诉你如何定义Java中的Student类和TreeSet集合,以及如何在其中存储和排序学生对象。
首先,我们可以定义一个名为Student的类,它包含三个属性:姓名 name、年龄 age 和班级 className。这个类的定义如下:
```java
public class Student implements Comparable<Student> {
private String name;
private int age;
private String className;
public Student(String name, int age, String className) {
this.name = name;
this.age = age;
this.className = className;
}
public String getName() {
return name;
}
public int getAge() {
return age;
}
public String getClassName() {
return className;
}
// 实现Comparable接口中的compareTo方法,用于排序
@Override
public int compareTo(Student o) {
return this.name.compareTo(o.name);
}
}
```
在这个类中,我们实现了一个Comparable接口,重写了其中的compareTo()方法,以便在TreeSet中按照姓名进行排序显示。接下来我们可以使用TreeSet来存储学生对象。代码如下:
```java
import java.util.TreeSet;
import java.io.*;
public class Main {
public static void main(String[] args){
// 创建一个TreeSet集合,用于存储学生对象
TreeSet<Student> students = new TreeSet<>();
try {
// 创建一个文件读取器
BufferedReader reader = new BufferedReader(new FileReader("students.txt"));
String line;
// 从文件中逐行读取学生信息
while ((line = reader.readLine()) != null) {
String[] info = line.split(","); // 按照逗号分隔姓名、年龄、班级
String name = info[0].trim();
int age = Integer.parseInt(info[1].trim());
String className = info[2].trim();
Student student = new Student(name, age, className);
students.add(student); // 将学生对象加入到集合中
}
reader.close(); // 关闭文件读取器
} catch (IOException e) {
e.printStackTrace();
}
// 遍历集合中的学生对象,按照姓名顺序输出
for (Student student : students) {
System.out.println(student.getName() + ", " + student.getAge() + ", " + student.getClassName());
}
}
}
```
在这里,我们从一个名为"students.txt"的文件中读取学生信息,并将它们存储到TreeSet集合中。最后,我们遍历集合,并按照姓名的字母顺序输出学生信息。
虽然我不能像ChatGPT那样实时回答你的问题,但我希望这些代码可以帮到你,让你更好地了解如何在Java中定义类和集合,并实现类的排序。
阅读全文
相关推荐
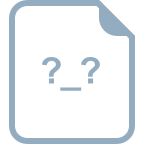
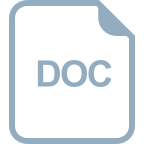
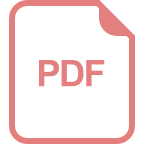














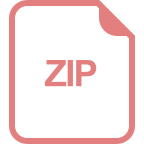
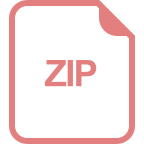