android篮球计分器两个界面两个代码
时间: 2023-12-14 12:03:40 浏览: 38
好的,下面是一个简单的 Android 篮球计分器应用程序的两个界面和代码实现:
1. 主界面
主界面包括两个篮球场地图,每个地图都有一个计分板和一个时间计时器。每个计分板应该显示两个队伍的分数、个人犯规和队伍犯规。时间计时器应该有开始、停止和重置按钮。此外,还应该有一个撤销按钮,以撤销上一次得分或犯规操作。
主界面的代码实现如下:
```
public class MainActivity extends AppCompatActivity implements View.OnClickListener {
private TextView tvTeam1Name, tvTeam2Name, tvTeam1Score, tvTeam2Score, tvTeam1PersonalFoul, tvTeam2PersonalFoul, tvTeam1TeamFoul, tvTeam2TeamFoul, tvTime;
private Button btnStart, btnStop, btnReset, btnUndo;
private ImageView ivCourt1, ivCourt2;
private int team1Score = 0, team2Score = 0;
private int team1PersonalFoul = 0, team2PersonalFoul = 0;
private int team1TeamFoul = 0, team2TeamFoul = 0;
private boolean isTimerStarted = false;
private boolean isTimerPaused = false;
private CountDownTimer countDownTimer;
private long timeLeftInMillis = 0;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
tvTeam1Name = findViewById(R.id.tv_team1_name);
tvTeam2Name = findViewById(R.id.tv_team2_name);
tvTeam1Score = findViewById(R.id.tv_team1_score);
tvTeam2Score = findViewById(R.id.tv_team2_score);
tvTeam1PersonalFoul = findViewById(R.id.tv_team1_personal_foul);
tvTeam2PersonalFoul = findViewById(R.id.tv_team2_personal_foul);
tvTeam1TeamFoul = findViewById(R.id.tv_team1_team_foul);
tvTeam2TeamFoul = findViewById(R.id.tv_team2_team_foul);
tvTime = findViewById(R.id.tv_time);
btnStart = findViewById(R.id.btn_start);
btnStop = findViewById(R.id.btn_stop);
btnReset = findViewById(R.id.btn_reset);
btnUndo = findViewById(R.id.btn_undo);
ivCourt1 = findViewById(R.id.iv_court1);
ivCourt2 = findViewById(R.id.iv_court2);
btnStart.setOnClickListener(this);
btnStop.setOnClickListener(this);
btnReset.setOnClickListener(this);
btnUndo.setOnClickListener(this);
// 初始化计时器
timeLeftInMillis = 60000;
updateCountDownText();
// 初始化撤销按钮状态
btnUndo.setEnabled(false);
}
@Override
public void onClick(View v) {
switch (v.getId()) {
case R.id.btn_start:
startTimer();
break;
case R.id.btn_stop:
pauseTimer();
break;
case R.id.btn_reset:
resetTimer();
break;
case R.id.btn_undo:
undoLastAction();
break;
}
}
// 开始计时器
private void startTimer() {
if (!isTimerStarted) {
countDownTimer = new CountDownTimer(timeLeftInMillis, 1000) {
@Override
public void onTick(long millisUntilFinished) {
timeLeftInMillis = millisUntilFinished;
updateCountDownText();
}
@Override
public void onFinish() {
isTimerStarted = false;
isTimerPaused = false;
btnStart.setEnabled(true);
btnStop.setEnabled(false);
btnReset.setEnabled(true);
btnUndo.setEnabled(false);
timeLeftInMillis = 0;
updateCountDownText();
Toast.makeText(MainActivity.this, "比赛结束!", Toast.LENGTH_SHORT).show();
}
}.start();
isTimerStarted = true;
isTimerPaused = false;
btnStart.setEnabled(false);
btnStop.setEnabled(true);
btnReset.setEnabled(false);
btnUndo.setEnabled(true);
} else if (isTimerPaused) {
countDownTimer.resume();
isTimerPaused = false;
btnStart.setEnabled(false);
btnStop.setEnabled(true);
btnReset.setEnabled(false);
btnUndo.setEnabled(true);
}
}
// 暂停计时器
private void pauseTimer() {
if (isTimerStarted && !isTimerPaused) {
countDownTimer.pause();
isTimerPaused = true;
btnStart.setEnabled(true);
btnStop.setEnabled(false);
btnReset.setEnabled(true);
btnUndo.setEnabled(true);
}
}
// 重置计时器
private void resetTimer() {
if (isTimerStarted || isTimerPaused) {
countDownTimer.cancel();
isTimerStarted = false;
isTimerPaused = false;
btnStart.setEnabled(true);
btnStop.setEnabled(false);
btnReset.setEnabled(false);
btnUndo.setEnabled(false);
timeLeftInMillis = 60000;
updateCountDownText();
}
}
// 撤销上一次得分或犯规操作
private void undoLastAction() {
// TODO: 撤销上一次得分或犯规操作的代码
}
// 更新倒计时文本
private void updateCountDownText() {
int minutes = (int) (timeLeftInMillis / 1000) / 60;
int seconds = (int) (timeLeftInMillis / 1000) % 60;
String timeLeftFormatted = String.format(Locale.getDefault(), "%02d:%02d", minutes, seconds);
tvTime.setText(timeLeftFormatted);
}
}
```
2. 设置界面
设置界面包括设置球队名称、队长、颜色和背景图像等选项。此外,还应该有一个计时器设置选项,允许用户设置每个节次的持续时间和休息时间。
设置界面的代码实现如下:
```
public class SettingsActivity extends AppCompatActivity implements View.OnClickListener {
private EditText etTeam1Name, etTeam2Name, etTeam1Captain, etTeam2Captain, etTeam1Color, etTeam2Color;
private ImageView ivTeam1Background, ivTeam2Background;
private Button btnSave;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_settings);
etTeam1Name = findViewById(R.id.et_team1_name);
etTeam2Name = findViewById(R.id.et_team2_name);
etTeam1Captain = findViewById(R.id.et_team1_captain);
etTeam2Captain = findViewById(R.id.et_team2_captain);
etTeam1Color = findViewById(R.id.et_team1_color);
etTeam2Color = findViewById(R.id.et_team2_color);
ivTeam1Background = findViewById(R.id.iv_team1_background);
ivTeam2Background = findViewById(R.id.iv_team2_background);
btnSave = findViewById(R.id.btn_save);
btnSave.setOnClickListener(this);
}
@Override
public void onClick(View v) {
switch (v.getId()) {
case R.id.btn_save:
saveSettings();
break;
}
}
// 保存设置
private void saveSettings() {
String team1Name = etTeam1Name.getText().toString();
String team2Name = etTeam2Name.getText().toString();
String team1Captain = etTeam1Captain.getText().toString();
String team2Captain = etTeam2Captain.getText().toString();
String team1Color = etTeam1Color.getText().toString();
String team2Color = etTeam2Color.getText().toString();
// TODO: 保存设置的代码
}
}
```
以上是一个简单的 Android 篮球计分器应用程序的两个界面和代码实现,你可以根据自己的需求进行更改和优化。
相关推荐
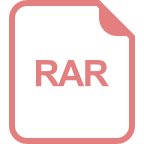
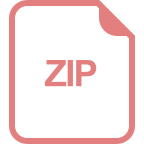
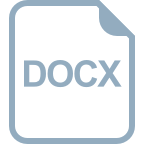



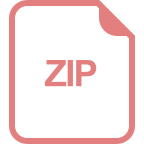
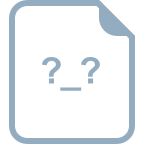
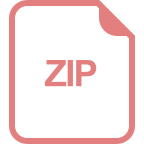