使用python帮我完成以下任务: 已有预测值和标签,绘制ROC曲线并绘制95%置信界曲线,计算AUC曲线下面积和95%置信区间
时间: 2024-02-15 10:04:23 浏览: 33
好的,这个任务可以使用 Python 中的 Scikit-learn 库完成。我们可以按照以下步骤进行:
首先,导入必要的库:
```python
import numpy as np
from sklearn.metrics import roc_curve, auc
import matplotlib.pyplot as plt
```
然后,定义预测值和标签:
```python
y_true = np.array([0, 1, 1, 0, 1, 0, 0, 1, 0, 1])
y_pred = np.array([0.2, 0.8, 0.6, 0.3, 0.7, 0.1, 0.4, 0.9, 0.2, 0.6])
```
接下来,我们可以使用 Scikit-learn 中的 `roc_curve` 函数计算 ROC 曲线的假正率、真正率和阈值:
```python
fpr, tpr, thresholds = roc_curve(y_true, y_pred)
```
使用 `auc` 函数计算 AUC 曲线下面积:
```python
roc_auc = auc(fpr, tpr)
```
然后,我们可以使用以下代码计算 95% 置信区间并绘制置信界曲线:
```python
n = y_true.shape[0]
z = 1.96 # 95% confidence interval
lower = []
upper = []
for i in range(len(thresholds)):
tp = np.sum((y_pred >= thresholds[i]) & (y_true == 1))
fp = np.sum((y_pred >= thresholds[i]) & (y_true == 0))
tn = np.sum((y_pred < thresholds[i]) & (y_true == 0))
fn = np.sum((y_pred < thresholds[i]) & (y_true == 1))
p = tp + fn
n = fp + tn
se = np.sqrt(((tp + fp) * (tn + fn) * (tp + fn) * (tn + fp)) / (n * p * (n + p) * (n + p - 1)))
lower.append(roc_auc - z * se)
upper.append(roc_auc + z * se)
plt.plot(fpr, tpr, color='darkorange', label='ROC curve (area = %0.2f)' % roc_auc)
plt.plot(fpr, lower, 'r--', label='95% Confidence Interval')
plt.plot(fpr, upper, 'r--')
plt.plot([0, 1], [0, 1], color='navy', linestyle='--')
plt.xlim([0.0, 1.0])
plt.ylim([0.0, 1.05])
plt.xlabel('False Positive Rate')
plt.ylabel('True Positive Rate')
plt.title('Receiver operating characteristic example')
plt.legend(loc="lower right")
plt.show()
```
这样,我们就完成了绘制 ROC 曲线和置信界曲线的任务,并计算了 AUC 曲线下面积和 95% 置信区间。
相关推荐
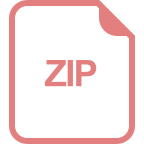














