某百货公司为了促销,采用购物打折的优惠办法。顾客一次购物折扣如下表所示。编写应用程序,由用户输入购物价值,计算并输出优惠价,结果保留1位小数。
时间: 2024-03-21 20:43:54 浏览: 179
好的,根据你的要求,以下是一个可以实现此功能的 Python 程序:
```python
price = float(input("请输入购物价值:"))
if price >= 200:
discount = price * 0.8
elif price >= 100:
discount = price * 0.9
else:
discount = price
print("优惠价为:{:.1f}".format(discount))
```
程序首先让用户输入购物价值,然后根据表格中的条件进行判断,计算出相应的优惠价,并使用字符串格式化将结果输出。注意,这里使用了 `float()` 函数将用户输入的字符串转换为浮点数,以便进行数值计算。
相关问题
某百货公司为了促销,采用购物打折的办法。1000元以上者,按九五折优惠;2000元以上者,按九折优惠;3000元以上者,按八五折优惠;5000元以上者,按八折优惠。编写程序,输入购物款数,计算并输出优惠价。python
在Python中,你可以创建一个函数来处理这个折扣策略。下面是一个简单的示例,该程序会根据用户输入的购物金额计算相应的优惠价格:
```python
def calculate_discount(price):
if price >= 5000:
discount_rate = 0.80 # 八折
elif price >= 3000:
discount_rate = 0.85 # 八五折
elif price >= 2000:
discount_rate = 0.90 # 九折
else: # 即1000元以上者
discount_rate = 0.95 # 九五折
discounted_price = price * discount_rate
return discounted_price
# 用户输入购物金额
shopping_amount = float(input("请输入您的购物总额(元):"))
discounted_amount = calculate_discount(shopping_amount)
print(f"您的优惠价是:{discounted_amount}元")
某百货公司为了促销,采用购物打折的优惠方法,每位顾客一次购物: 在1000元以上者,按9.5折优惠; 在2000以上者,按9折优惠; 在3000以上者,按8.5折优惠; 在5000以上者,按8折优惠; 编写程序,购物款数,计算并输出优惠价。答案保留两位小数
这是一个简单的条件分支处理问题。可以编写一个函数,接收顾客的购物总额作为输入,然后根据不同的折扣规则计算出优惠后的价格。下面是一个Python示例:
```python
def calculate_discount(price):
if price >= 5000:
discount = 0.8
elif price >= 3000:
discount = 0.85
elif price >= 2000:
discount = 0.9
else:
# 如果在1000元以上,但不到2000元,折扣为9.5%
discount = 0.95
# 计算优惠价并四舍五入到两位小数
discounted_price = price * discount
return round(discounted_price, 2)
# 用户输入购物款数,例如
shopping_amount = float(input("请输入您的购物金额(元):"))
discounted_amount = calculate_discount(shopping_amount)
print(f"您的优惠价为:{discounted_amount}元")
```
在这个程序中,首先检查购物金额是否满足某个折扣条件,然后应用相应的折扣率,最后计算并返回优惠价。
阅读全文
相关推荐
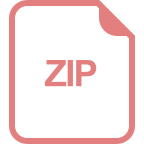
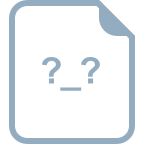
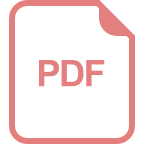













