c语言统计字符串中字母个数
时间: 2023-05-08 17:55:45 浏览: 133
要统计字符串中字母个数,可以先遍历整个字符串,逐个字符判断是否是字母,如果是,则字母个数加1,最后输出字母个数即可。
具体实现可以使用C语言中的字符串处理函数来完成,如下面的代码示例:
```c
#include <stdio.h>
#include <string.h>
#include <ctype.h>
int main() {
char str[100]; // 定义一个字符串
int len, i, letter_count = 0;
printf("请输入一个字符串:");
gets(str); // 输入字符串
len = strlen(str); // 获取字符串长度
for (i = 0; i < len; i++) {
if (isalpha(str[i])) { // 如果是字母
letter_count++; // 字母个数加1
}
}
printf("字母个数为:%d\n", letter_count);
return 0;
}
```
其中,isalpha()函数用来判断一个字符是否是字母,如果是返回非零值,否则返回0。在循环中遍历整个字符串,如果遇到字母,则字母个数加1。最后输出字母个数即可。
需要注意的是,在输入字符串时应尽量避免使用gets()函数,因为它存在安全性问题,容易造成缓冲区溢出。建议使用fgets()等安全的字符串输入函数来代替。
相关问题
c语言统计字符串中字母个数数字空格其他字符
好的,针对您的问题,我可以给您提供以下的 C 语言代码来统计一个字符串中字母、数字、空格和其他字符的个数:
```c
#include <stdio.h>
#include <ctype.h>
int main() {
char str[100];
int i, alphabets, digits, spaces, others;
alphabets = digits = spaces = others = 0;
printf("请输入一个字符串:");
fgets(str, sizeof(str), stdin);
for(i = 0; str[i] != '\0'; i++) {
if(isalpha(str[i])) {
alphabets++;
} else if(isdigit(str[i])) {
digits++;
} else if(str[i] == ' ') {
spaces++;
} else {
others++;
}
}
printf("字母个数:%d\n", alphabets);
printf("数字个数:%d\n", digits);
printf("空格个数:%d\n", spaces);
printf("其他字符个数:%d\n", others);
return 0;
}
```
这段代码中使用了 `isalpha()`、`isdigit()` 等函数来判断一个字符是否为字母或数字,同时也判断了空格和其他字符。您可以将输入的字符串改成您需要统计的字符串。
C语言统计字符串的字母个数和空格个数
要统计字符串的字母个数和空格个数,可以使用C语言的字符处理函数和循环来实现。下面是一个示例代码:
```c
#include <stdio.h>
#include <ctype.h>
int main() {
char str[100];
int letterCount = 0;
int spaceCount = 0;
printf("请输入一个字符串:");
fgets(str, sizeof(str), stdin);
for (int i = 0; str[i] != '\0'; i++) {
if (isalpha(str[i])) {
letterCount++;
} else if (isspace(str[i])) {
spaceCount++;
}
}
printf("字母个数:%d\n", letterCount);
printf("空格个数:%d\n", spaceCount);
return 0;
}
```
这段代码首先定义了一个字符数组 `str` 来存储输入的字符串,然后使用 `fgets` 函数从标准输入中读取字符串。接下来,通过一个循环遍历字符串中的每个字符,使用 `isalpha` 函数判断字符是否为字母,如果是,则字母计数器 `letterCount` 加一;使用 `isspace` 函数判断字符是否为空格,如果是,则空格计数器 `spaceCount` 加一。最后,输出字母个数和空格个数。
阅读全文
相关推荐
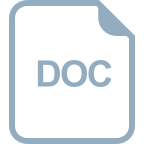
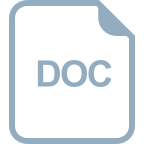
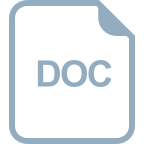
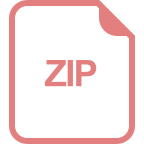












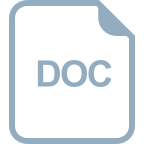